How To Build a Simple React To-Do App with React Hooks
Building a React To-Do app with React Hooks is a great way to learn how to use React Hooks in your projects.
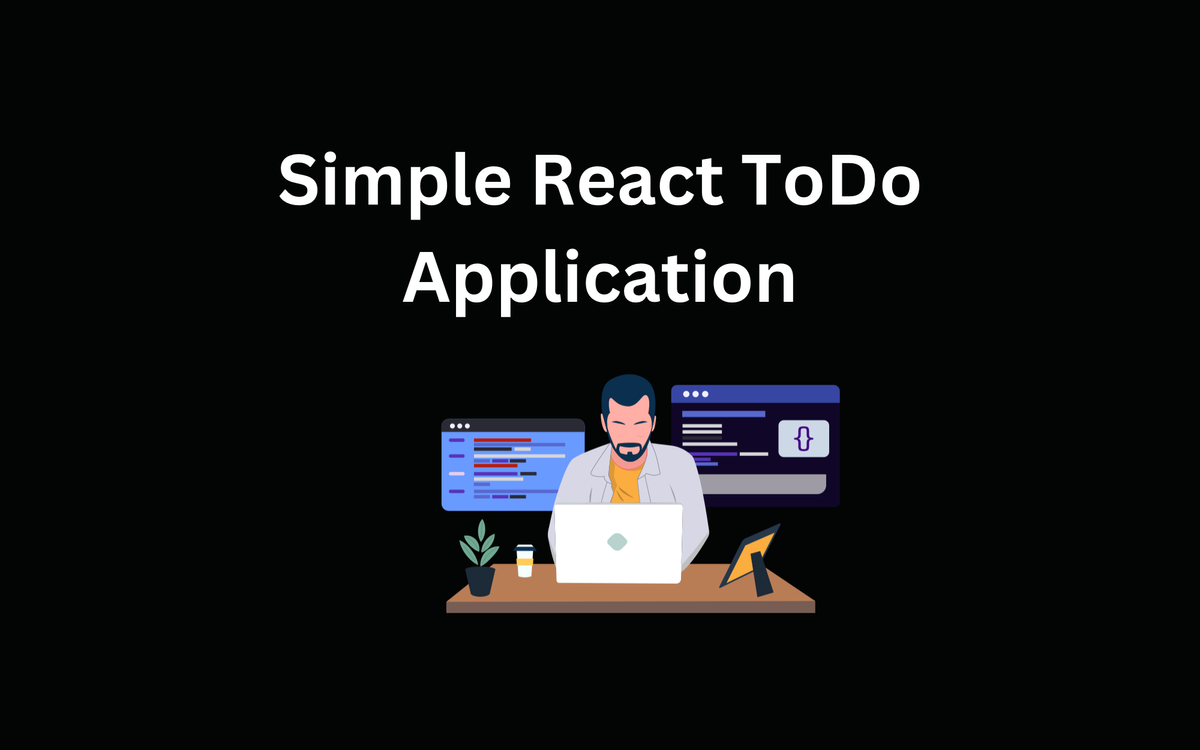
Building a React To-Do app with React Hooks is a great way to learn how to use React Hooks in your projects.
React Hooks are a new feature introduced in React 16.8 that allows you to use state and other React features without writing a class component.
Here are the steps to build a React To-Do app with React Hooks:
- Set up your React environment:
- Install Node.js and npm on your computer.
- Create a new React project using
create-react-app
.
2. Create a new file called TodoList.js
to define the component that will render the To-Do list.
3. Import the useState
hook from the React library:
import React, { useState } from 'react';
4. Define the initial state of your To-Do list using the useState hook:
const [todos, setTodos] = useState([]);
5. Create a new function called addTodo
that will add a new task to the list of To-Dos.
const addTodo = (text) => {
const newTodos = [...todos, { text }];
setTodos(newTodos);
}
6. Create a new component called TodoForm
that will render a form to add new tasks to the list. The component should contain a form with an input field and a button. When clicking the button, it should call the addTodo
function with the text entered in the input field.
function TodoForm({ addTodo }) {
const [value, setValue] = useState('');
const handleSubmit = e => {
e.preventDefault();
if (!value) return;
addTodo(value);
setValue('');
}
return (
<form onSubmit={handleSubmit}>
<input type="text" className="input" value={value} onChange={e => setValue(e.target.value)} />
<button type="submit" className="button">Add</button>
</form>
)
}
7. In the TodoList
component, render the TodoForm
component and pass the addTodo
function as a prop.
8. Render the list of To-Dos by mapping over the todos
state array and display each task in a list item.
function TodoList() {
const [todos, setTodos] = useState([]);
const addTodo = (text) => {
const newTodos = [...todos, { text }];
setTodos(newTodos);
}
return (
<div>
<TodoForm addTodo={addTodo} />
<ul>
{todos.map((todo, index) => (
<li key={index}>{todo.text}</li>
))}
</ul>
</div>
)
}
9. Finally, render the TodoList
component in your App.js
file.
That's it! You now have a fully functional To-Do app built with React Hooks. You can customize it further by adding functionality such as marking tasks as complete or deleting tasks from the list.