CodeChef - ATM
ATM problem from CodeChef.
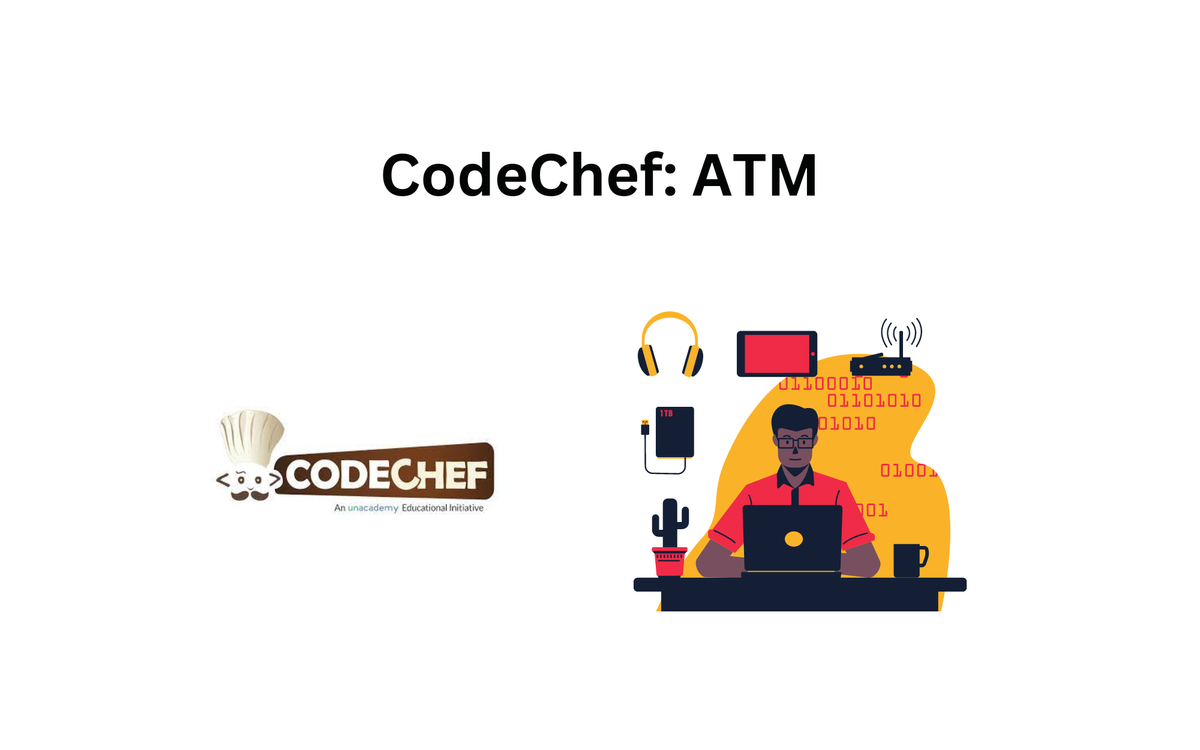
Problem
Pooja would like to withdraw X $US from an ATM. The cash machine will only accept the transaction if X is a multiple of 5, and Pooja's account balance has enough cash to perform the withdrawal transaction (including bank charges). For each successful withdrawal, the bank charges 0.50 $US.
Calculate Pooja's account balance after an attempted transaction.
Input Format
Each input contains 2 integers X
and Y
.
X
is the amount of cash that Pooja wishes to withdraw.
Y
is Pooja's initial account balance.
Output Format
Output the account balance after the attempted transaction, given as a number with two digits of precision. If there is not enough money in the account to complete the transaction, output the current bank balance.
Constraints
- 0 <= X <= 2000 - The amount of cash that pooja wishes to withdraw.
- 1 <= Y <= 2000 - With two digits of precision - pooja's initial account balance.
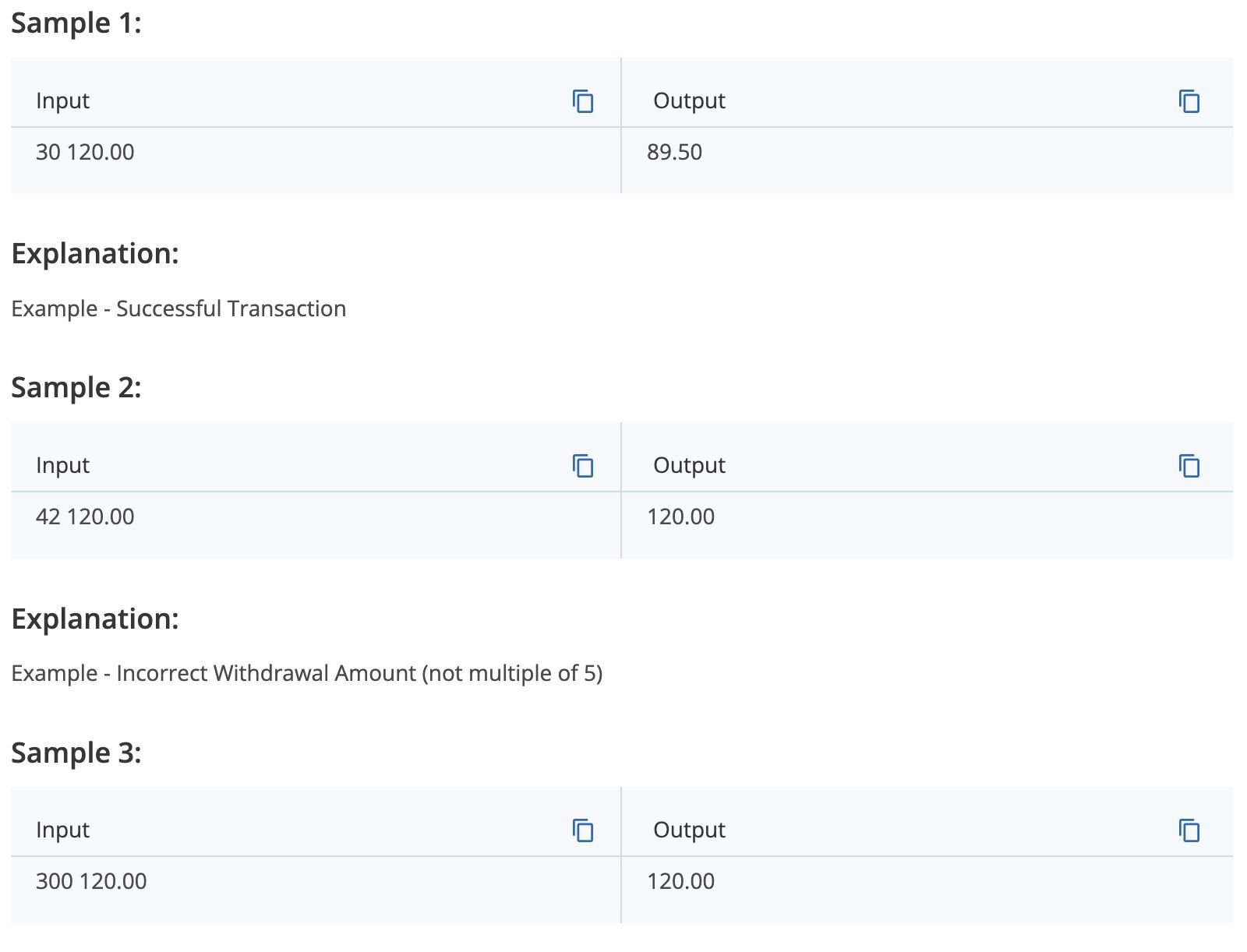
Solution in Java:
import java.util.*;
import java.io.*;
class Solution{
public static void main(String[] args) throws Exception{
InputStreamReader i = new InputStreamReader(System.in);
BufferedReader bf = new BufferedReader(i);
String[] in = bf.readLine().split(" ");
float n = Float.parseFloat(in[0]);
float f = Float.parseFloat(in[1]);
if(n%5==0 && f>=n+0.5f)
System.out.println(f-n-0.5f);
else{
System.out.println(f);
}
}
}