JavaScript ES6 Features
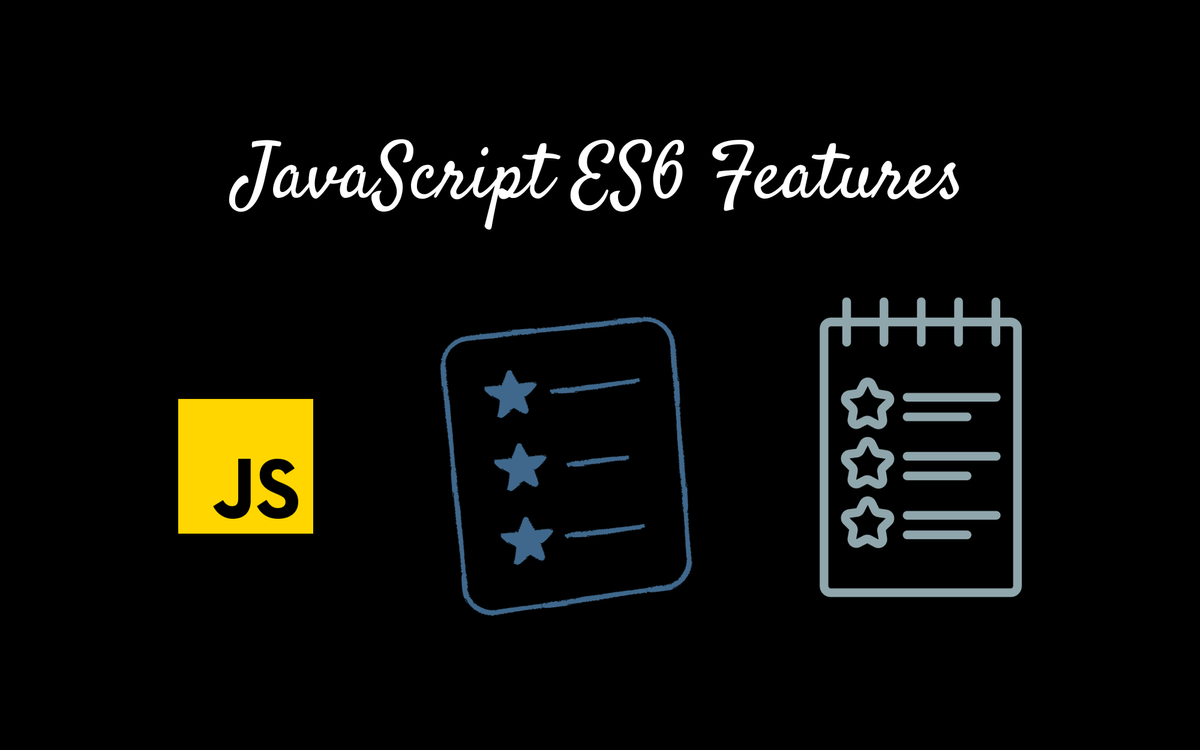
ES6 (ECMAScript 2015) introduced several important features to JavaScript. Here are some of the key features along with examples:
1. let and const
let
and const
are block-scoped variable declarations, which provide an alternative to var
.
// Using let
let x = 10;
x = 20; // Valid
// Using const
const y = 5;
y = 8; // Error: Assignment to constant variable
// Block scoping with let
if (true) {
let z = 30;
}
console.log(z); // Error: z is not defined
2. Arrow Functions
Arrow functions provide a concise syntax for writing function expressions.
// Traditional function
function add(x, y) {
return x + y;
}
// Arrow function
const add = (x, y) => x + y;
3. Template Literals
Template literals allow you to embed expressions inside strings.
const name = 'John';
const greeting = `Hello, ${name}!`;
4. Destructuring
Destructuring allows you to extract values from arrays or objects into separate variables.
// Destructuring an array
const [a, b] = [1, 2]; // a = 1, b = 2
// Destructuring an object
const { firstName, lastName } = { firstName: 'John', lastName: 'Doe' };
5. Spread and Rest Operators
The spread operator (...
) can be used to expand elements in an array or object. The rest operator is used to represent an indefinite number of arguments as an array.
// Spread operator
const arr1 = [1, 2, 3];
const arr2 = [...arr1, 4, 5]; // [1, 2, 3, 4, 5]
// Rest operator
function sum(...args) {
return args.reduce((total, current) => total + current, 0);
}
6. Object Shorthand
ES6 introduced a shorter syntax for defining object properties.
const name = 'John';
const age = 30;
const person = { name, age }; // { name: 'John', age: 30 }
7. Classes
ES6 introduced class syntax for defining objects and their behavior.
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
greet() {
return `Hello, my name is ${this.name} and I am ${this.age} years old.`;
}
}
const john = new Person('John', 30);
john.greet(); // "Hello, my name is John and I am 30 years old."
8. Modules
ES6 introduced a module system for organizing code into reusable files.
// In math.js
export function add(x, y) {
return x + y;
}
// In main.js
import { add } from './math.js';
console.log(add(2, 3)); // 5
9. Promises
Promises provide a way to handle asynchronous operations.
const fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched!');
}, 2000);
});
};
fetchData()
.then(data => console.log(data))
.catch(error => console.error(error));
10. Default Parameters
ES6 allows you to set default values for function parameters.
function greet(name = 'John') {
return `Hello, ${name}!`;
}
greet(); // "Hello, John!"
greet('Jane'); // "Hello, Jane!"
These are some of the key features introduced in ES6. They enhance the readability, maintainability, and expressiveness of JavaScript code. It's important for modern JavaScript developers to be familiar with these features as they are now standard in the language.