CodeChef - Enormous Input Test
Enormous Input Test problem from CodeChef.
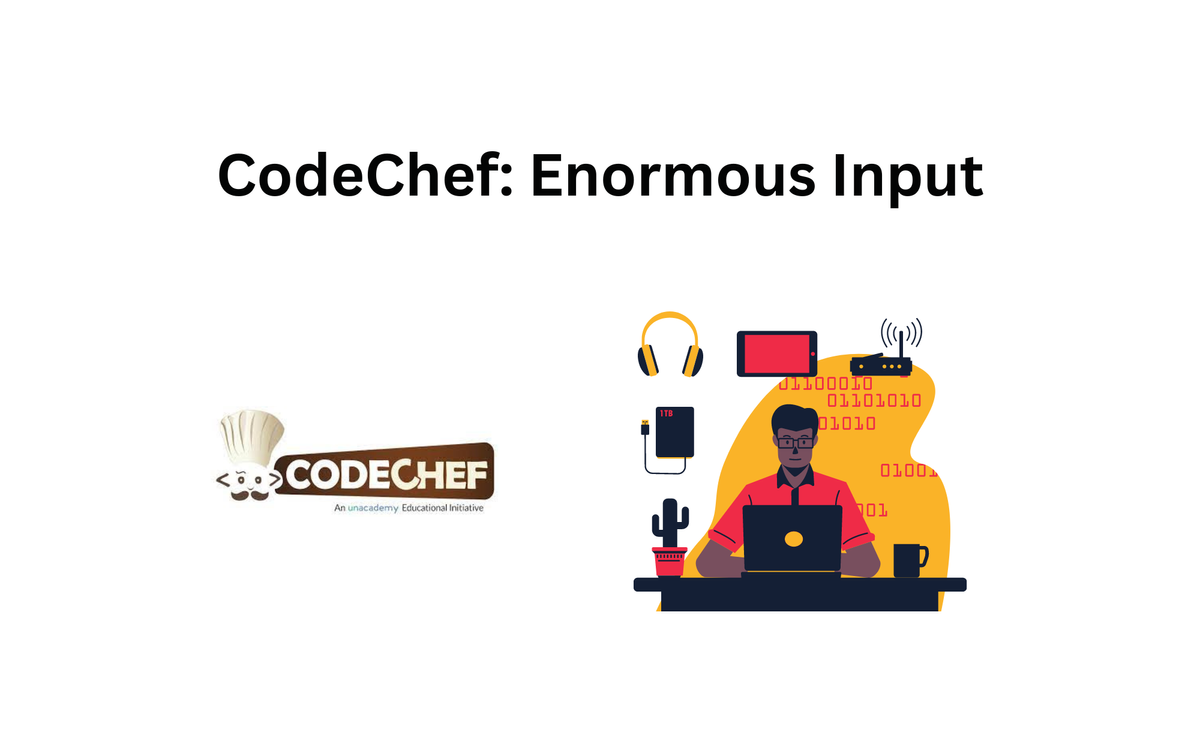
Problem
You are given N
integers. Find the count of numbers divisible by K
.
Input Format
The input begins with two positive integers N
, K
. The next N
lines contain one positive integer each denoted by Ai.
Output Format
Output a single number denoting how many integers are divisible by K
.
Constraints
- 1 <= N, K <= 107
- 1 <= Ai <= 109
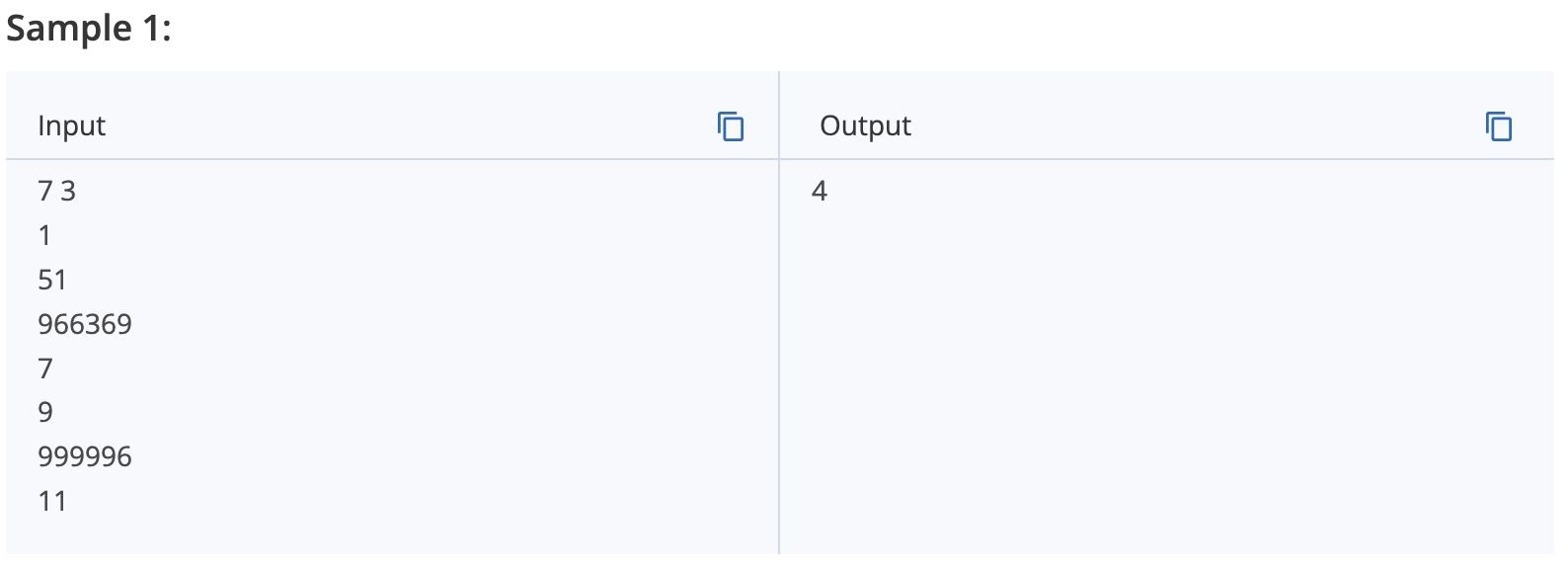
Solution in Java:
import java.io.OutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintWriter;
import java.util.StringTokenizer;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.InputStream;
// Remember that the class name should be "Main" and should be "public".
public class Main {
public static void main(String[] args) {
// System.in and System.out are input and output streams, respectively.
InputStream inputStream = System.in;
InputReader in = new InputReader(inputStream);
int n = in.nextInt();
int k = in.nextInt();
int ans = 0;
for (int i = 0; i < n; i++) {
int x = in.nextInt();
if (x % k == 0) {
ans++;
}
}
System.out.println(ans);
}
static class InputReader {
public BufferedReader reader;
public StringTokenizer tokenizer;
public InputReader(InputStream stream) {
reader = new BufferedReader(new InputStreamReader(stream), 32768);
tokenizer = null;
}
public String next() {
while (tokenizer == null || !tokenizer.hasMoreTokens()) {
try {
tokenizer = new StringTokenizer(reader.readLine());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return tokenizer.nextToken();
}
public int nextInt() {
return Integer.parseInt(next());
}
}
}