CodeChef - Find Remainder
Find Remainder problem from CodeChef.
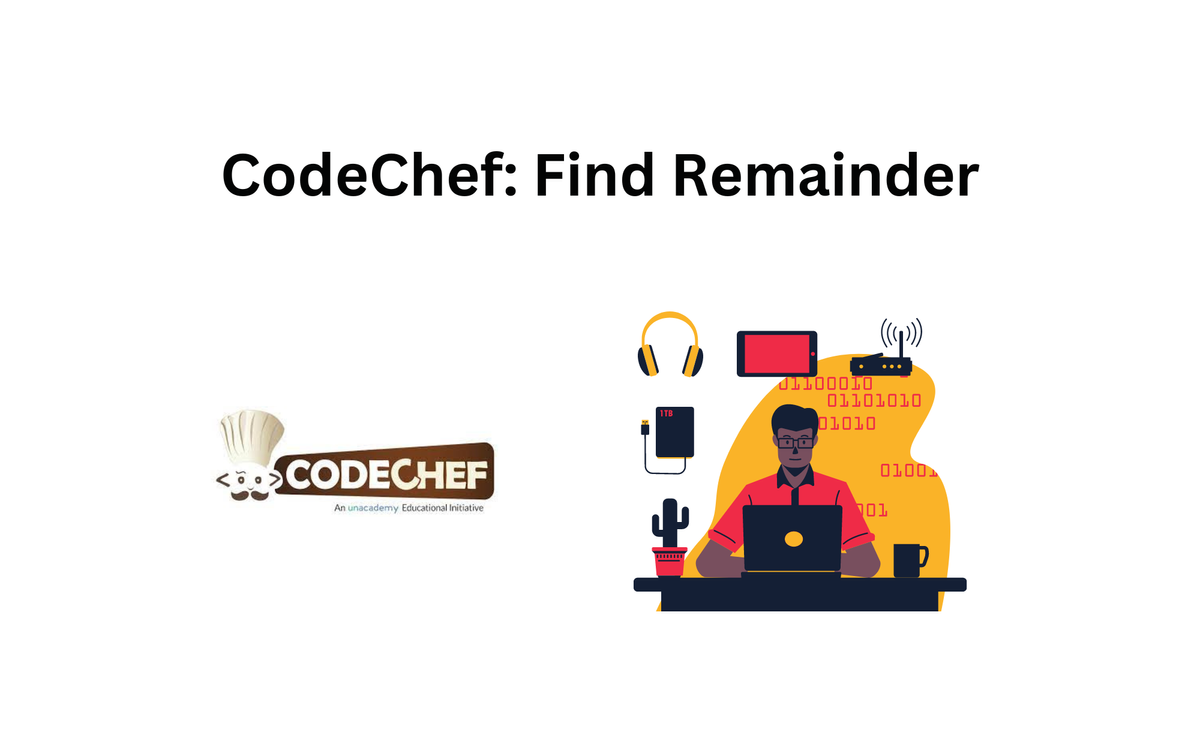
Problem
Write a program to find the remainder when an integer A
is divided by an integer B
.
Input
The first line contains an integer T
, the total number of test cases. Then T
lines follow, each line contains two Integers A
and B
.
Output
For each test case, find the remainder when A
is divided by B
, and display it in a new line.
Constraints
- 1 <= T <= 1000
- 1 <= A, B <= 10000
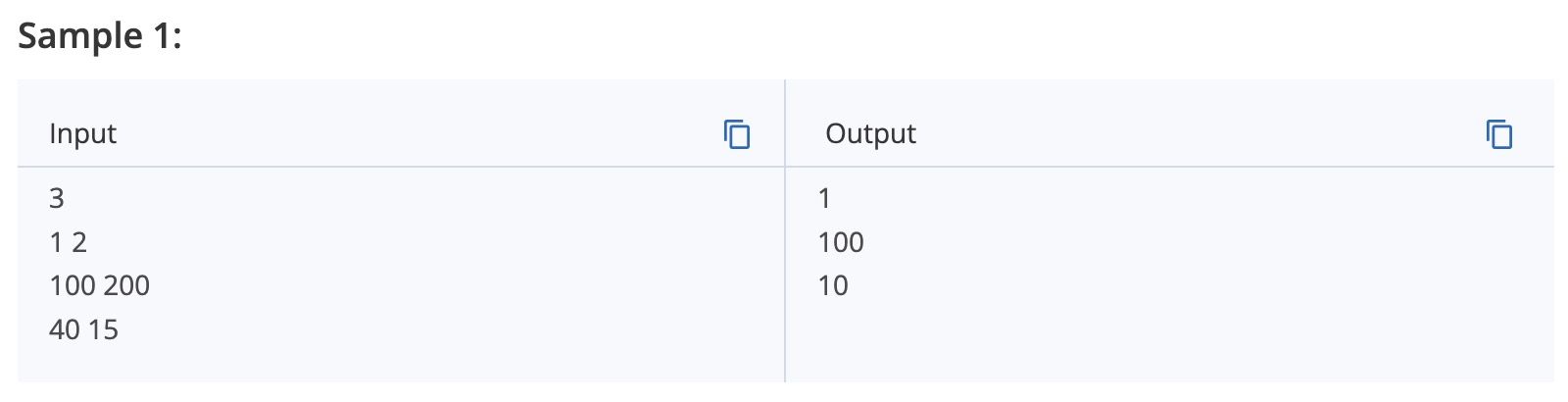
Solution in Java:
import java.util.*;
class Remainder
{
public static void main(String args[])
{
Scanner s = new Scanner(System.in);
int t = s.nextInt();
while(t-->0)
{
int a = s.nextInt();
int b = s.nextInt();
System.out.println(a%b);
}
}
}