CodeChef - Hoop Jump
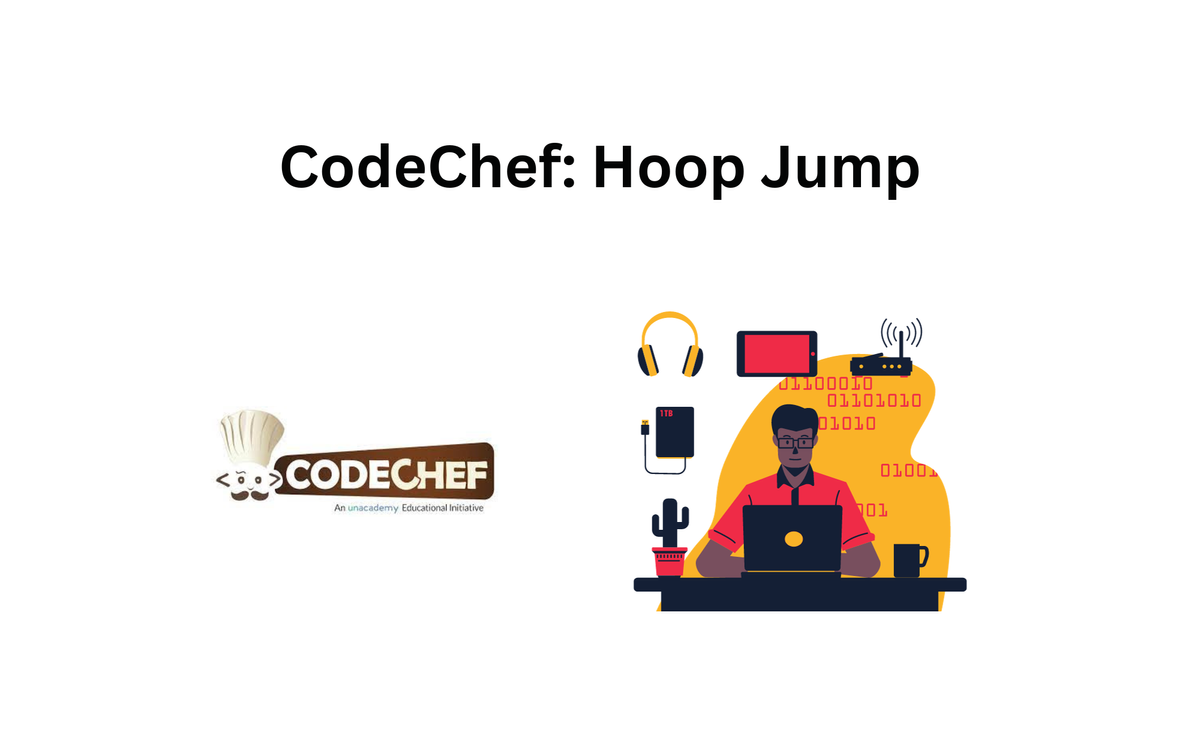
You and your friend are playing a game with hoops. There are N hoops (where N is odd) in a row. You jump into hoop 1, and your friend jumps into hoop N. Then you jump into hoop 2, and after that, your friend jumps into hoop N−1, and so on.
The process ends when someone cannot make the next jump because the hoop is occupied by the other person. Find the last hoop that will be jumped into.
Input
- The first line contains an integer T, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, a single integer N.
Output
For each testcase, output in a single line the answer to the problem.
Constraints
- 1 ≤ T ≤ 105
- 1 ≤ N < 2⋅105
- N is odd
Subtasks
Subtask #1 (100 points): original constraints
Sample 1:
Input Output
2 1
1 2
3
Explanation:
Test Case 1: Since there is only 11 hoop, that's the only one to be jumped into.
Test Case 2: The first player jumps into hoop 11. The second player jumps into hoop 33 and finally the first player jumps into hoop 22. Then the second player cannot make another jump, so the process stops.
import java.util.Scanner;
class HoopJump {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int T = sc.nextInt();
while (T-- > 0) {
int N = sc.nextInt();
System.out.println((N / 2) + 1);
}
sc.close();
}
}