CodeChef: ID and Ship Solution
CodeChef: ID and Ship Solution
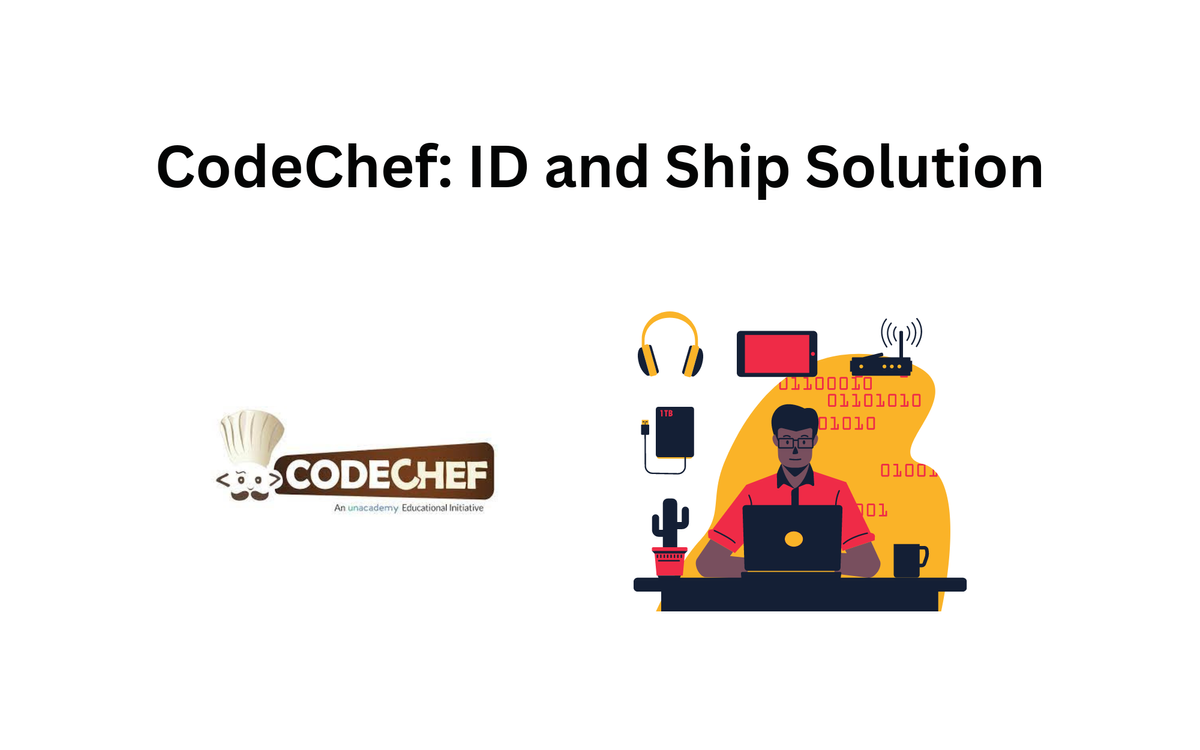
Problem
Write a program that takes in a letter class ID of a ship and displays the equivalent string class description of the given ID. Use the table below.
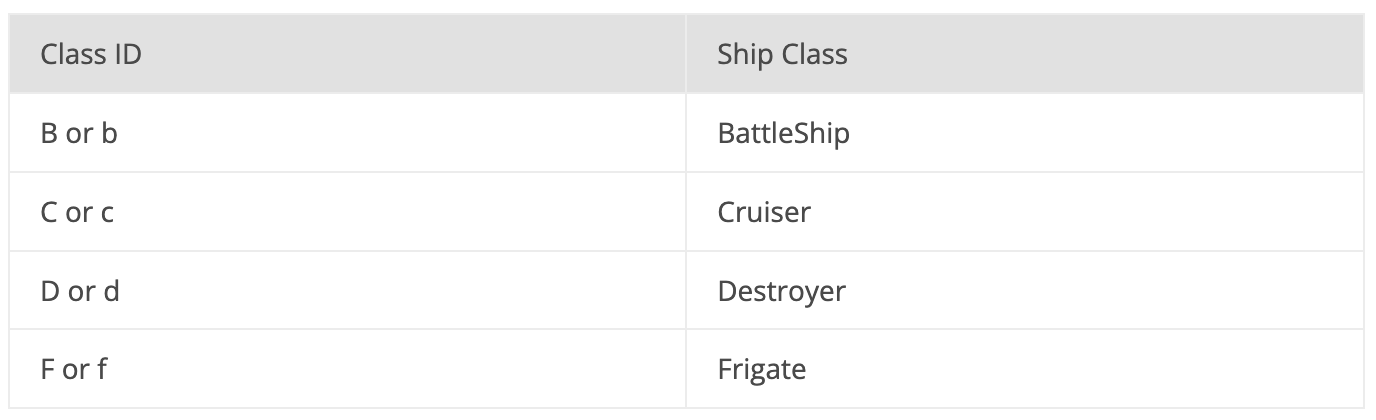
Input Format
The first line contains an integer T, the total number of test cases. Then T lines follow, each line contains a character.
Output Format
For each test case, display the Ship Class depending on the ID, in a new line.
Constraints
1 ≤ T ≤ 1000
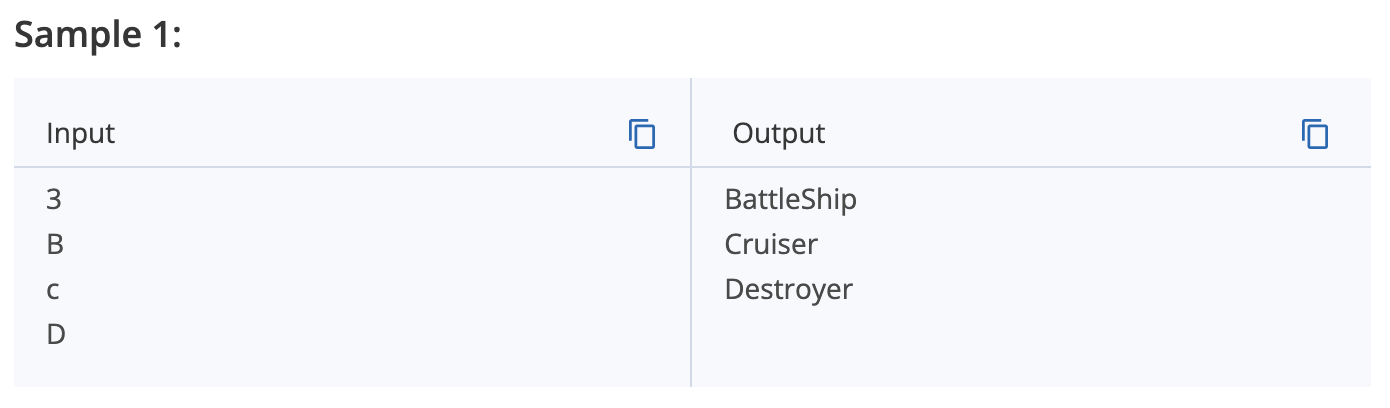
Solution
import java.util.*;
import java.lang.*;
import java.io.*;
/* Name of the class has to be "Main" only if the class is public. */
class Codechef
{
public static void main (String[] args) throws java.lang.Exception
{
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for(int i = 0; i < n; i++) {
String str=sc.next();
if(str.equalsIgnoreCase("b")) {
System.out.println("BattleShip");
}
else if(str.equalsIgnoreCase("c"))
{
System.out.println("Cruiser");
}
else if(str.equalsIgnoreCase("d")) {
System.out.println("Destroyer");
}
else if(str.equalsIgnoreCase("f")) {
System.out.println("Frigate");
}
else {
break;
}
}
}
}