CodeChef - Reverse The Number
Reverse The Number problem from CodeChef.
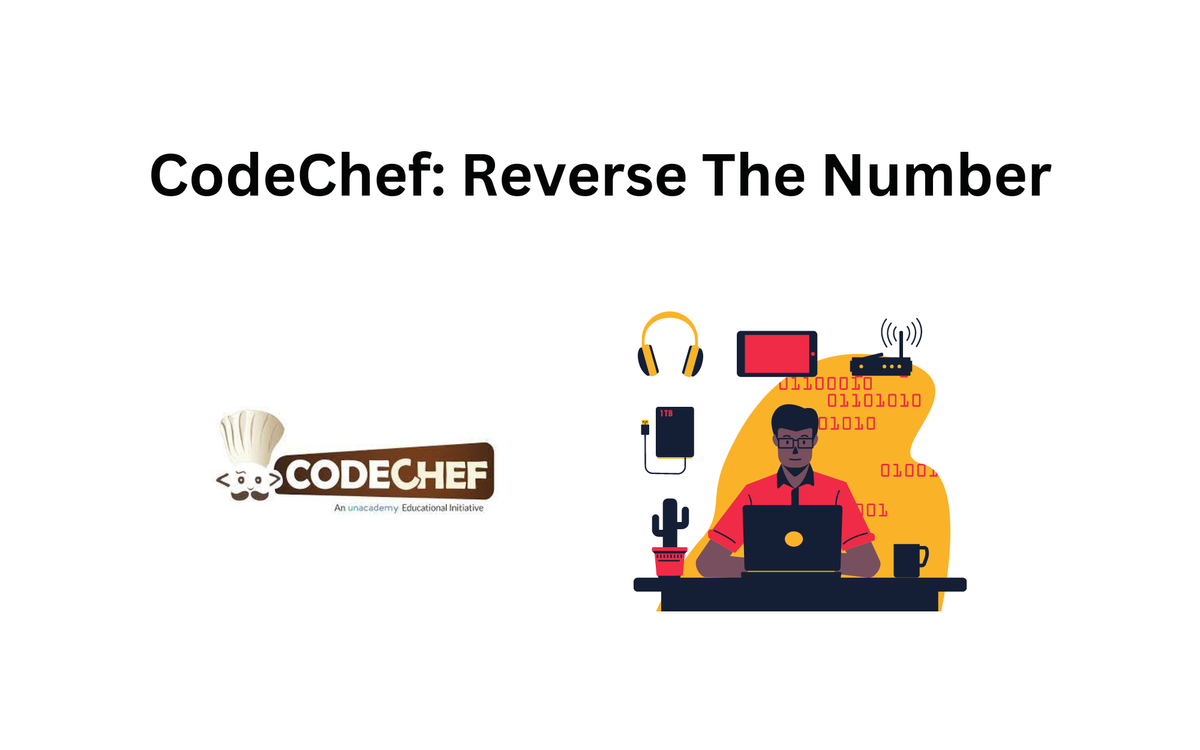
Problem
Given an Integer N
, write a program to reverse it.
Input
The first line contains an integer T
, the total number of test cases. Then follow T
lines, each line contains an integer N
.
Output
For each test case, display the reverse of the given number N
, in a new line.
Constraints
- 1 <= T <= 1000
- 1 <= N <= 1000000
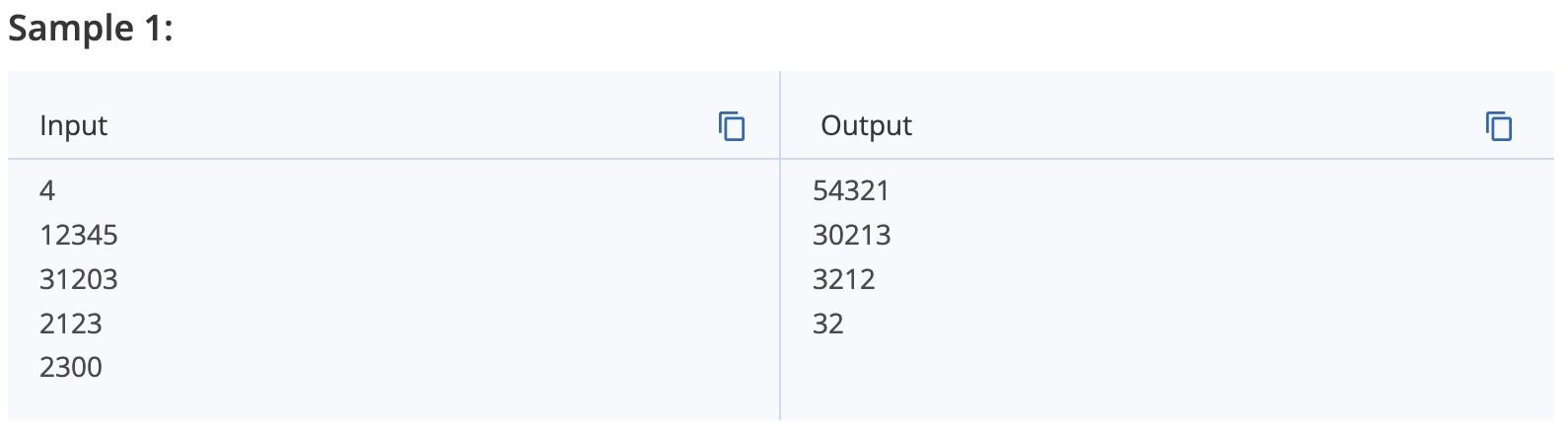
Solution in Java:
import java.util.*;
import java.lang.*;
import java.io.*;
class Codechef2{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
int T = sc.nextInt();
while(T-- > 0){
long l = sc.nextLong();
long val = 0;
while( l != 0){
long v = l % 10;
val = val * 10 + v;
l /= 10;
}
System.out.println(val);
}
sc.close();
}
}