Codechef - Weight Balance
Weight Balance problem from CodeChef.
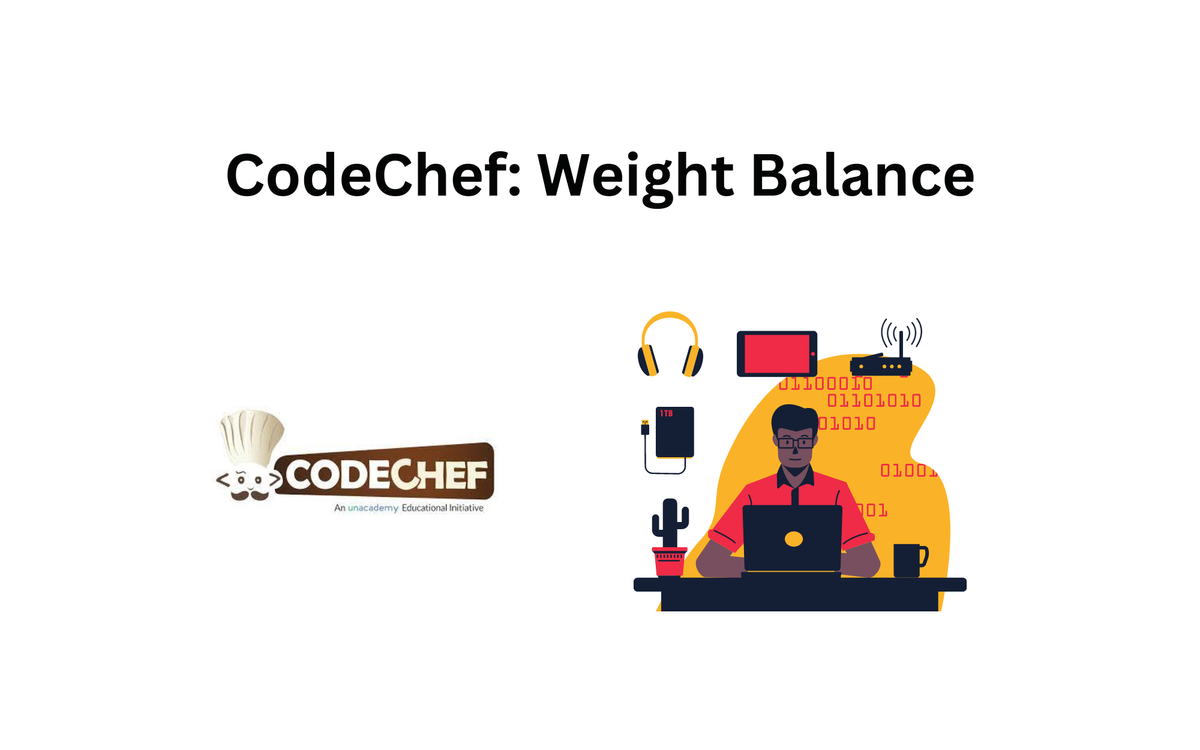
Problem
No play and eating all day make your belly fat. This happened to Chef during the lockdown. His weight before the lockdown was w
1
kg (measured on the most accurate hospital machine) and after M
months of lockdown, when he measured his weight at home (on a regular scale, which can be inaccurate), he got the result that his weight was w
2
kg (2>w2>w1
).
Scientific research on all growing kids shows that their weights increase by a value between x
1
and x
2
kg (inclusive) per month, but not necessarily the same value each month. Chef assumes that he is a growing kid. Tell him whether his home scale could be giving the correct results
Scientific research on all growing kids shows that their weights increase by a value between x
1
and x
2
kg (inclusive) per month, but not necessarily the same value each month. Chef assumes that he is a growing kid. Tell him whether his home scale could be giving correct results.
Input
- The first line of the input contains a single integer
T
denoting the number of test cases. The description ofT
test cases follows. - The first and only line of each test case contains five space-separated integers
w
1
,w
2
,x
1
,x
2
andM
.
Output
For each test case, print a single line containing the integer 1 if the result shown by the scale can be correct or 0 if it cannot.
Constraints
- 1 <= T <= 105
- 1 <= w1 < w2 <= 100
- 1 <= x1 <= x2 <= 10
- 1 <= M <= 10
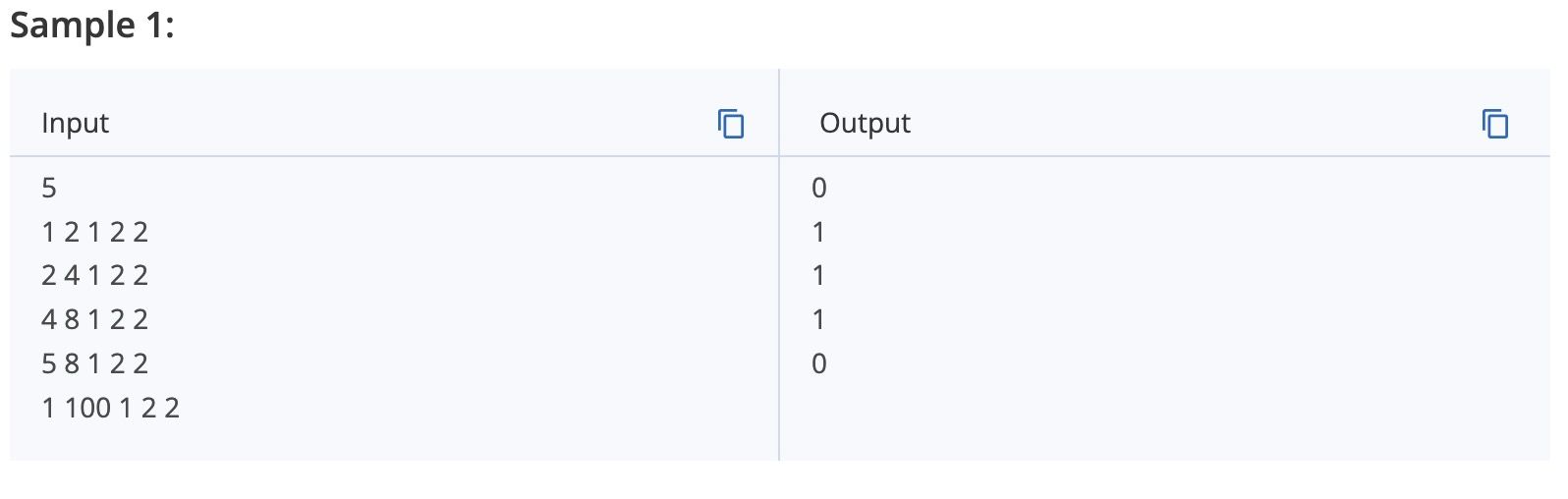
Explanation:
Example case 1: Since the increase in Chef's weight is 2 - 1 = 1 kg and that is less than the minimum possible increase of 1.2 = 2 kg, the scale must be faulty.
Example case 2: Since the increase in Chef's weight is 4 - 2 = 2 kg, which is equal to the minimum possible increase of 1.2 = 2 kg, the scale is showing correct results.
Example case 3: Since the increase in Chef's weight is 8 - 4 = 4 kg, which is equal to the maximum possible increase of 2.2 = 4 kg, the scale is showing correct results.
Example case 4: Since the increase in Chef's weight is 8 - 5 = 3 kg, which lies in the interval [1.2, 2.2]kg, the scale is showing correct results.
Example case 5: Since the increase in Chef's weight is 100 - 1 = 99 kg and that is more than the maximum possible increase 2.2 = 4 kg, the weight balance must be faulty.
Solution :
import java.util.*;
class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int T = sc.nextInt();
while(T-- > 0) {
int w1 = sc.nextInt();
int w2 = sc.nextInt();
int x1 = sc.nextInt();
int x2 = sc.nextInt();
int m = sc.nextInt();
int ans = (w2 - w1 >= x1 * m && w2 - w1 <= x2 * m ? 1 : 0);
System.out.println(ans);
}
sc.close();
}
}