Java Assignment Operators
Java assignment operators provide a shorthand way of modifying the value of a variable by performing some operation on it and then assigning the result back to the variable.
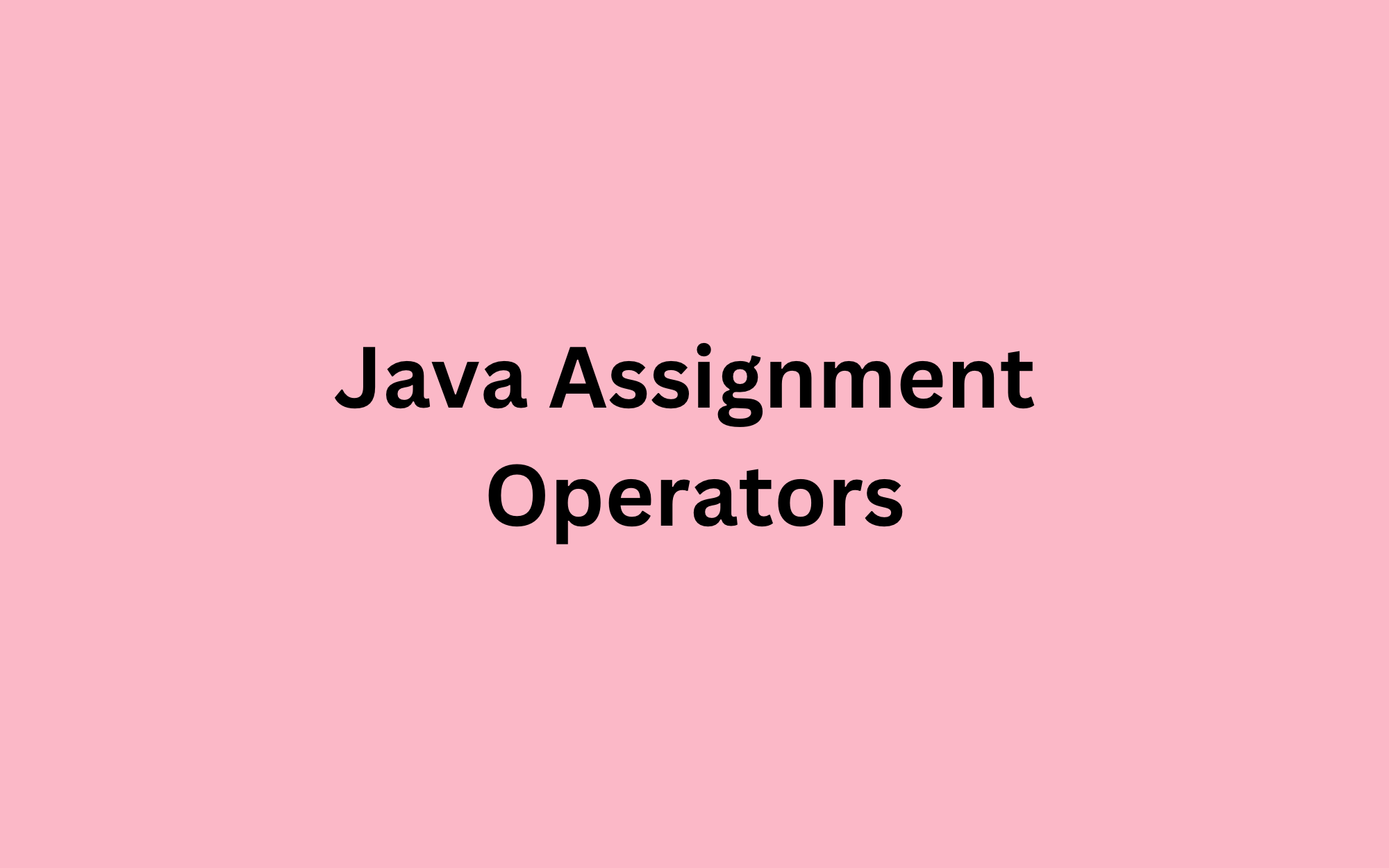
Introduction
In Java, assignment operators are used to assigning values to variables. They provide a shorthand way of modifying the value of a variable by performing some operation on it and then assigning the result back to the variable.
The most common assignment operator is the simple assignment operator =
, which assigns the value on the right-hand side to the variable on the left-hand side. However, Java also provides several other assignment operators, such as +=
, -=
, *=
, /=
, and %=
, which perform arithmetic operations and assign the result to the variable. These operators are useful in simplifying code and making it more concise.
Assignment operators
In Java, assignment operators are used to assigning values to variables. They allow you to modify the value of a variable by performing some operation on it and then assigning the result back to the variable.
Here are the common assignment operators in Java:
=
: The simple assignment operator assigns the value on the right-hand side to the variable on the left-hand side.
Example:
int x = 10;
2. +=
: The addition assignment operator adds the value on the right-hand side to the variable on the left-hand side.
Example:
int x = 10;
x += 5; // x is now 15
3. -=
: The subtraction assignment operator subtracts the value on the right-hand side from the variable on the left-hand side.
Example:
int x = 10;
x -= 5; // x is now 5
4. *=
: The multiplication assignment operator multiplies the value on the right-hand side by the variable on the left-hand side.
Example:
int x = 10;
x *= 5; // x is now 50
5. /=
: The division assignment operator divides the value on the left-hand side by the value on the right-hand side.
Example:
int x = 10;
x /= 2; // x is now 5
6. %=
: The modulus assignment operator divides the value on the left-hand side by the value on the right-hand side and returns the remainder.
Example:
int x = 10;
x %= 3; // x is now 1
Note that all of these assignment operators can be combined with other operators, such as the increment and decrement operators.
Conclusion
In conclusion, assignment operators are an important part of Java programming language, as they allow developers to assign values to variables and perform arithmetic operations in a concise and efficient way.
Java provides several assignment operators such as +=
, -=
, *=
, /=
, and %=
, which helps in making code more readable and reducing the amount of code required to perform certain tasks.
Understanding how to use assignment operators is crucial for writing efficient and effective Java programs.