Java Break And Continue
In Java, the "break" and "continue" keywords are used to control the flow of execution within loops.
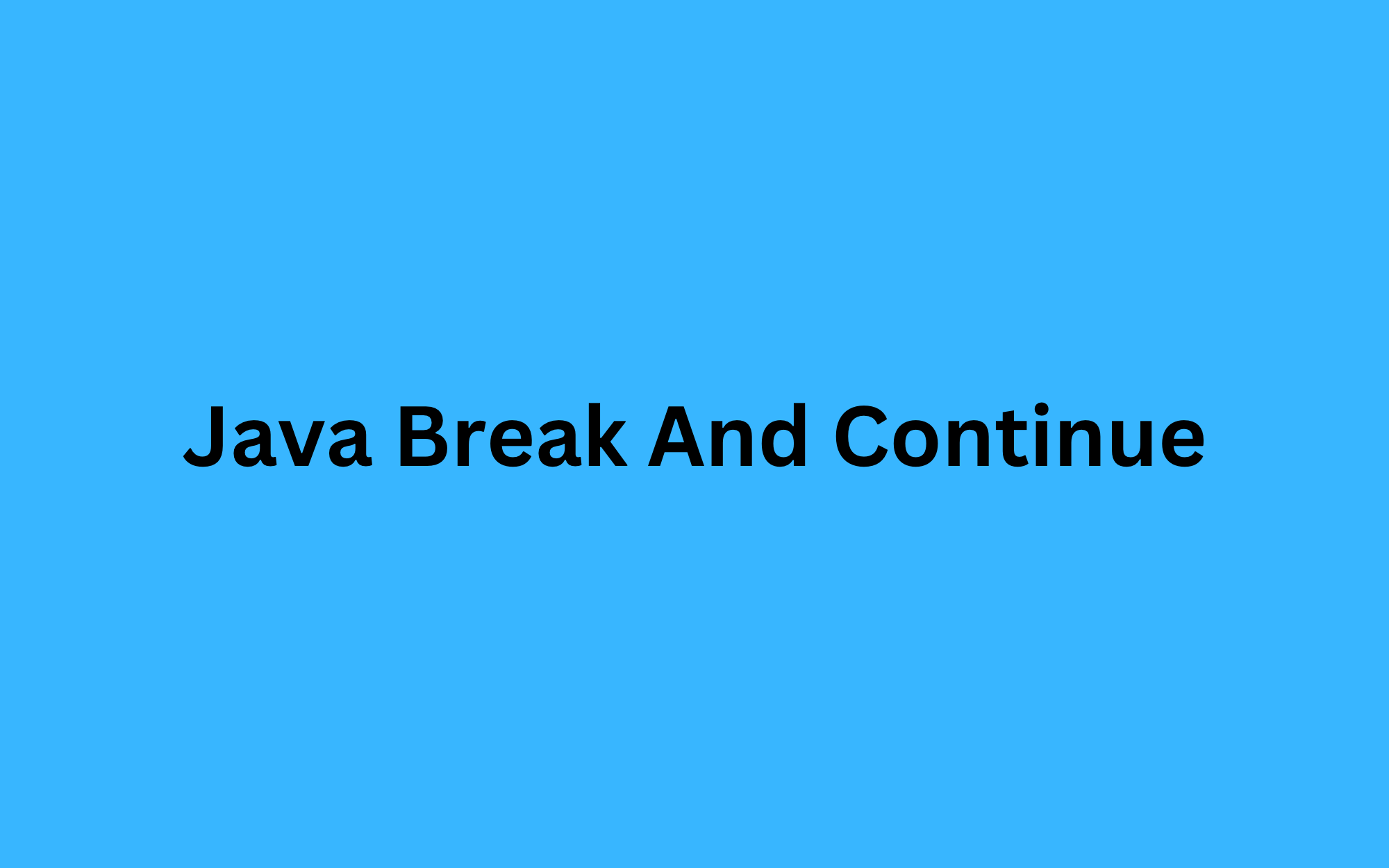
Introduction
In Java, the break
and continue
keywords are used to control the flow of execution within loops.
The "break" statement is used to terminate the loop prematurely, while the "continue" statement is used to skip over the current iteration and move on to the next iteration of the loop.
These statements can be used to add more flexibility and control to your loops, allowing you to customize the behavior of your code based on specific conditions or requirements.
Syntax and declaration
The break
and continue
statements are control statements in Java that are used to alter the normal flow of execution in a loop or switch statement.
The syntax for break
statement is:
break;
This statement is used to terminate the execution of a loop or switch statement. When the break
statement is encountered inside a loop or switch statement, the control is transferred to the next statement after the loop or switch.
The syntax for continue
statement is:
continue;
This statement is used to skip the current iteration of a loop and move on to the next iteration. When the continue
statement is encountered inside a loop, the control jumps to the beginning of the loop and starts the next iteration.
Both break
and continue
statements can be used inside for
, while
, and do-while
loops as well as switch statements.
It's important to use these statements judiciously, as they can make the code more complex and harder to read if used excessively or improperly. However, they can also be very useful in certain situations where it's necessary to alter the normal flow of execution in a loop or switch statement.
Examples
Here are some examples of break
and continue
statements in Java:
Example 1
Using break
statement in a for
loop:
for (int i = 1; i <= 10; i++) {
if (i == 6) {
break;
}
System.out.println(i);
}
In this example, the loop will iterate from 1 to 10. When i
becomes 6, the break
statement is encountered and the loop is terminated. Therefore, the output of this code will be:
1
2
3
4
5
Example 2
Using continue
statement in a while
loop
int i = 0;
while (i < 10) {
i++;
if (i == 5) {
continue;
}
System.out.println(i);
}
In this example, the loop will iterate from 1 to 10. When i
becomes 5, the continue
statement is encountered and the current iteration is skipped. Therefore, the output of this code will be:
1
2
3
4
6
7
8
9
10
Example 3
Using break
statement in a switch
statement
int day = 5;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
case 4:
System.out.println("Thursday");
break;
case 5:
System.out.println("Friday");
break;
default:
System.out.println("Weekend");
break;
}
In this example, the switch
statement will print the name of the day corresponding to the value of the day
variable. When day
is 5 (which corresponds to Friday), the code will print "Friday" and then exit the switch
statement because of the break
statement. If there was no break
statement in the case 5
block, the code would continue executing and print "Weekend" as well.
Conclusion
In conclusion, break
and continue
are important control flow statements in Java that allow you to alter the flow of a loop or switch statement.
The break
statement can be used to immediately terminate a loop or switch statement and continue with the code following it. This can be useful when you need to exit a loop early or when you want to break out of a switch statement.
The continue
statement can be used to skip to the next iteration of a loop, ignoring any remaining code in the current iteration. This can be useful when you want to skip over certain iterations of a loop based on a condition.
Both statements can help you write more efficient and readable code by allowing you to control the flow of your program. However, they should be used judiciously and only when necessary, as overuse can make your code harder to understand and maintain.