Java Do-While Loop
In Java, the do-while loop is a variation of the while loop that guarantees the loop body will execute at least once, even if the condition is initially false.
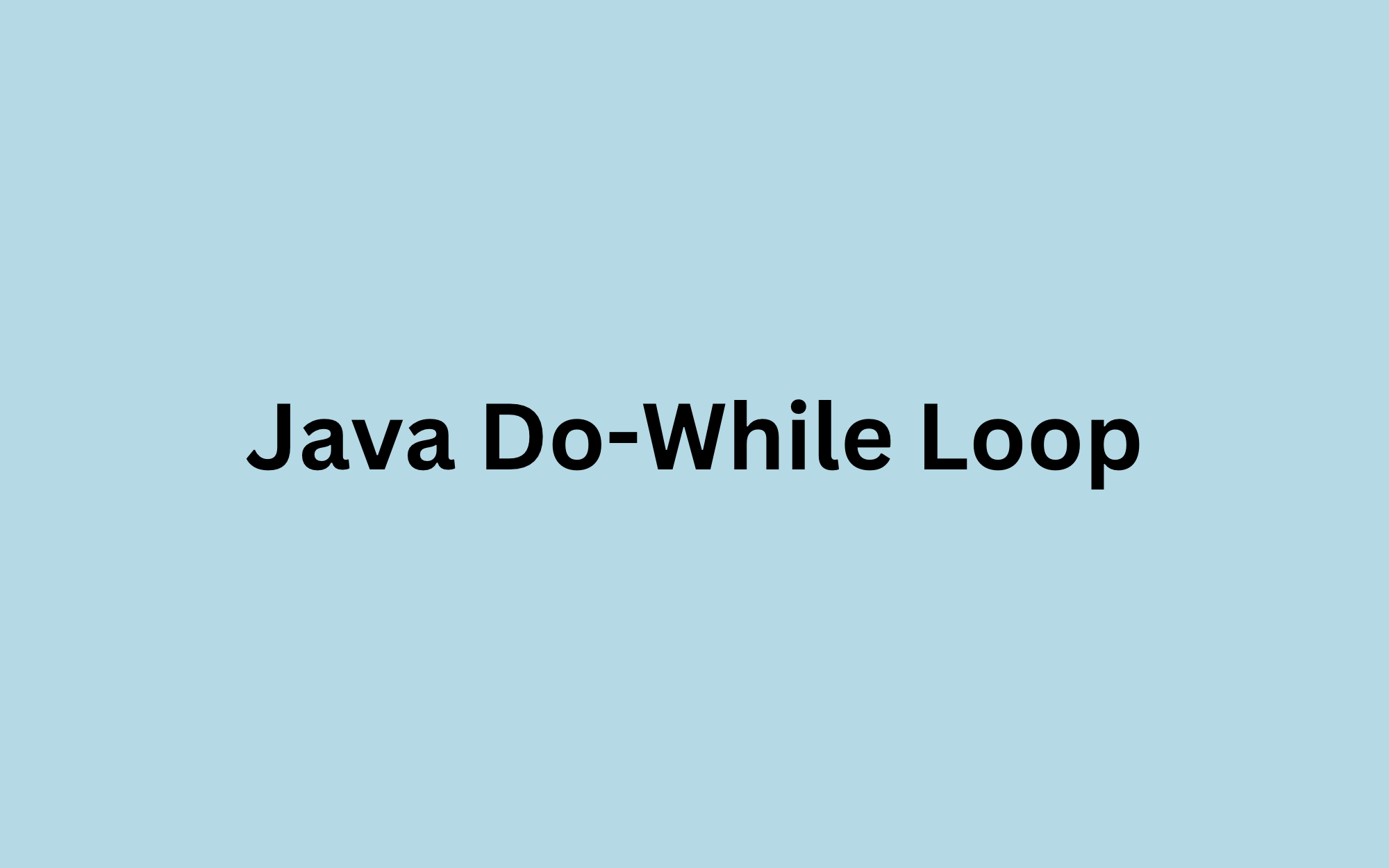
Introduction
In Java, the do-while
loop is a variation of the while
loop that guarantees the loop body will execute at least once, even if the condition is initially false.
The do-while
loop first executes the loop body and then checks the condition. If the condition is true, the loop body is executed again. This process repeats until the condition becomes false. Because of this, the do-while
loop is useful in situations where the loop body must be executed at least once, regardless of the initial condition.
Syntax and declaration
The syntax for the do-while
loop in Java is as follows:
do {
// code to be executed
} while (condition);
The loop body is enclosed in curly braces and contains the code that will be executed at least once. The condition is checked after the loop body has executed. If the condition is true, the loop body is executed again. If the condition is false, the loop terminates and control passes to the next statement after the loop.
Note that the condition is checked at the end of the loop body, which is why the loop body always executes at least once, even if the condition is initially false.
Here are some basic to advanced examples of do-while
loops in Java.
Basic do-while
int i = 0;
do {
System.out.println("Iteration " + i);
i++;
} while (i < 5);
In this example, the loop body is executed once before checking the condition. The loop will continue to execute as long as i
is less than 5.
Using a sentinel value
Scanner scanner = new Scanner(System.in);
String input;
do {
System.out.print("Enter a command (or 'quit' to exit): ");
input = scanner.nextLine();
System.out.println("You entered: " + input);
} while (!input.equals("quit"));
In this example, the loop will continue to execute until the user enters the word "quit". The loop prompts the user to enter a command and then prints out the user's input. The loop will continue to run until the user enters the word "quit", at which point the loop will terminate.
Nested do-while loops
int i = 0;
do {
int j = 0;
do {
System.out.print("*");
j++;
} while (j < 5);
System.out.println();
i++;
} while (i < 5);
In this example, the do-while
loop is nested inside another do-while
loop. The outer loop iterates five times, and the inner loop iterates five times for each iteration of the outer loop. The result is a block of asterisks printed on the console. This technique can be useful for complex tasks that require multiple levels of iteration.
Do-while loop with a break statement
int i = 0;
do {
if (i == 5) {
break;
}
System.out.println("Iteration " + i);
i++;
} while (true);
In this example, the do-while
loop is designed to iterate infinitely until i
reaches 5. The loop includes a conditional statement that checks if i
is equal to 5, and if so, it uses a break
statement to exit the loop. This technique can be useful in situations where it's not possible to know ahead of time how many iterations will be required.
Overall, do-while
loops provide a lot of flexibility and control when it comes to program flow. They allow you to execute code at least once and then check a condition to see if the loop should continue or not. This makes them particularly useful in situations where you need to ensure that a particular block of code executes at least once, regardless of the initial condition.
Conclusion
In conclusion, the do-while
loop in Java is a variation of the while
loop that executes the loop body at least once, even if the condition is initially false. The loop will continue to execute as long as the condition is true, and will terminate once the condition becomes false.
The do-while
loop is useful in situations where you need to ensure that a particular block of code executes at least once, regardless of the initial condition. Additionally, do-while
loops can be used to create nested loops, read input from the user, and create loops with a break
statement.
With their flexibility and control, do-while
loops are a valuable tool for any Java programmer.