Java If Statements
In Java, if statements are used to control the flow of a program by executing certain blocks of code only if a particular condition is met.
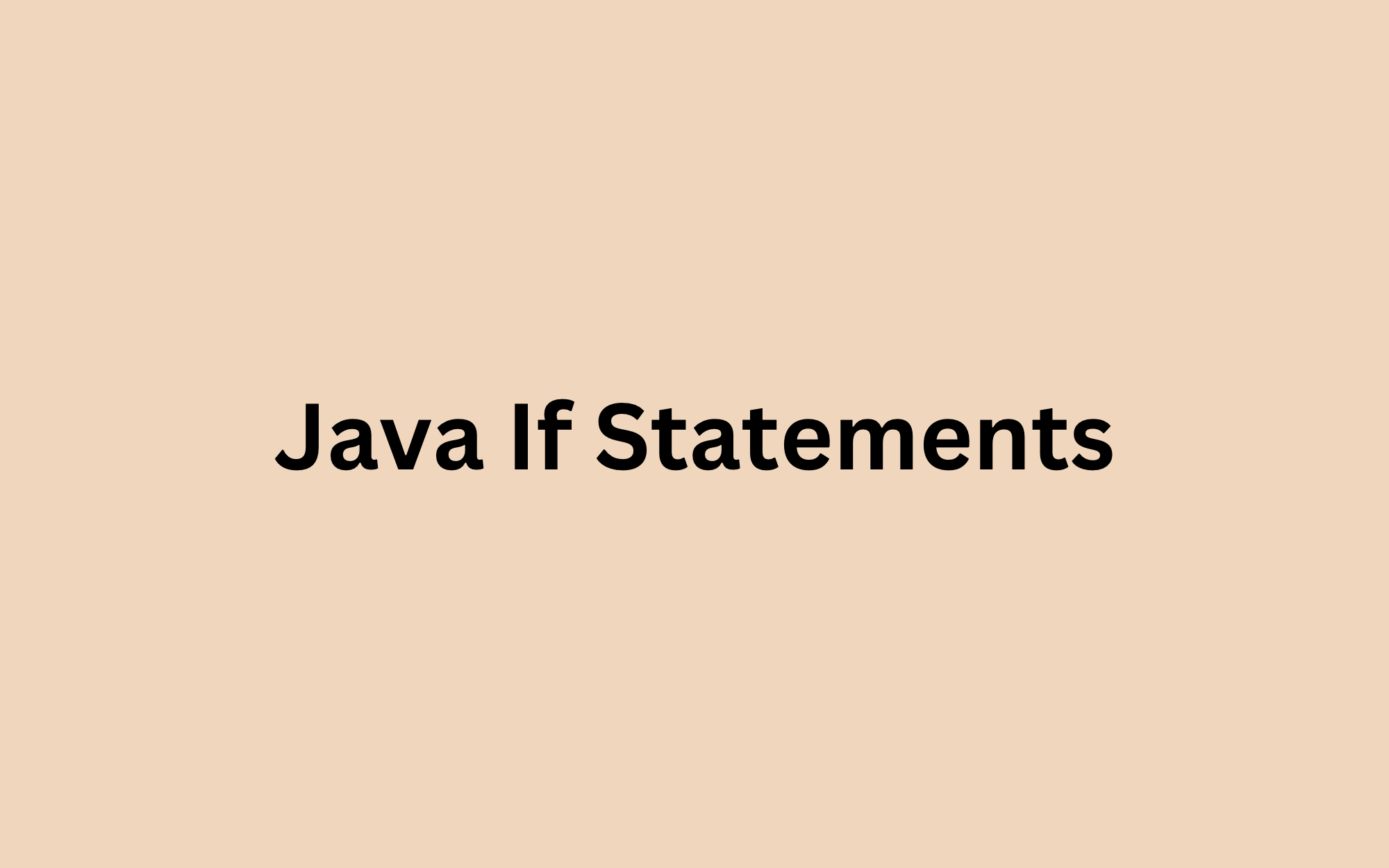
Introduction
In Java, if
statements are used to control the flow of a program by executing certain blocks of code only if a particular condition is met.
An if
statement consists of a condition followed by a block of code that is executed only if the condition is true. if
statements can also include else
clauses, which specify blocks of code to be executed if the condition is false.
if
statements are a fundamental part of programming in Java, and they are used extensively in a wide variety of applications.
Syntax and declaration
The basic syntax for an if
statement in Java is as follows.
if (condition) {
// code to be executed if condition is true
}
The condition
is an expression that is evaluated to either true
or false
. If the condition is true
, the block of code inside the braces { }
will be executed. If the condition is false
, the block of code will be skipped and the program will continue to the next line of code after the if
statement.
An if
statement can also include an else
clause, which is executed if the condition is false
. The syntax for an if-else
statement is:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
In this case, if the condition is true
, the block of code inside the first set of braces { }
will be executed. If the condition is false
, the block of code inside the second set of braces { }
will be executed.
Example snippet
// Simple if statement
if (x > 5) {
System.out.println("x is greater than 5");
}
// If-else statement
if (x > 5) {
System.out.println("x is greater than 5");
} else {
System.out.println("x is less than or equal to 5");
}
// Nested if statement
if (x > 5) {
if (x < 10) {
System.out.println("x is between 5 and 10");
}
}
// If-else if-else statement
if (x > 10) {
System.out.println("x is greater than 10");
} else if (x > 5) {
System.out.println("x is between 5 and 10");
} else {
System.out.println("x is less than or equal to 5");
}
// Ternary operator
int y = (x > 5) ? 10 : 5;
Types
In Java, there are several types of if
statements that can be used to control the flow of a program.
These include:
- Simple if statement
- If-else statement
- Nested if statement
- If-else if-else statement
- Ternary operator
Simple if statement
This is the basic if
statement that we covered in the previous answer. It executes a block of code if a certain condition is true.
Here's an example of a simple if
statement in Java:
int x = 10;
if (x > 5) {
System.out.println("x is greater than 5");
}
In this example, the if
statement checks whether the value of the variable x
is greater than 5. If the condition is true, which it is in this case, the block of code inside the braces { }
is executed, which prints the message "x is greater than 5" to the console.
If the condition were false, the block of code inside the braces would be skipped, and the program would move on to the next line of code after the if
statement.
If-else statement
This statement executes a block of code if the condition is true, and a different block of code if the condition is false.
Here's an example of an if-else
statement in Java:
int x = 3;
if (x > 5) {
System.out.println("x is greater than 5");
} else {
System.out.println("x is less than or equal to 5");
}
In this example, the if
statement checks whether the value of the variable x
is greater than 5. If the condition is true, the block of code inside the first set of braces { }
is executed, which prints the message "x is greater than 5" to the console. If the condition is false, the block of code inside the second set of braces { }
is executed, which prints the message "x is less than or equal to 5" to the console.
Since the value of x
is 3 in this example, the condition x > 5
is false, and the program executes the code inside the else
block, printing "x is less than or equal to 5" to the console.
Nested-if statement
This is an if
statement inside another if
statement. It can be used to check multiple conditions.
Here's an example of a nested if
statement in Java:
int x = 10;
int y = 5;
if (x > 5) {
System.out.println("x is greater than 5");
if (y > 2) {
System.out.println("y is greater than 2");
}
} else {
System.out.println("x is less than or equal to 5");
}
In this example, the outer if
statement checks whether the value of the variable x
is greater than 5. If the condition is true, the block of code inside the outer set of braces { }
is executed, which prints the message "x is greater than 5" to the console.
Inside this block, there is another if
statement that checks whether the value of the variable y
is greater than 2. If this condition is true, the block of code inside the inner set of braces { }
is executed, which prints the message "y is greater than 2" to the console.
Since both conditions are true in this example, the program executes both blocks of code, printing "x is greater than 5" and "y is greater than 2" to the console.
If-else if-else statement
This statement checks multiple conditions in a sequence, executing a different block of code for each condition.
Here's an example of an if-else if-else
statement in Java:
int x = 7;
if (x < 5) {
System.out.println("x is less than 5");
} else if (x > 10) {
System.out.println("x is greater than 10");
} else {
System.out.println("x is between 5 and 10");
}
In this example, the if
statement checks whether the value of the variable x
is less than 5. If the condition is true, the block of code inside the first set of braces { }
is executed, which prints the message "x is less than 5" to the console. If the condition is false, the program moves on to the next condition.
The second condition checks whether the value of x
is greater than 10. If this condition is true, the block of code inside the second set of braces { }
is executed, which prints the message "x is greater than 10" to the console. If this condition is also false, the program moves on to the final else
block.
The final else
block is executed only if all previous conditions are false. In this case, the block of code inside the third set of braces { }
is executed, which prints the message "x is between 5 and 10" to the console.
Since the value of x
is 7 in this example, the third condition x > 10
is false, but the first condition x < 5
is also false. Therefore, the program executes the code inside the final else
block, printing "x is between 5 and 10" to the console.
Ternary operator
This is a shorthand if-else
statement that can be used to assign a value to a variable based on a condition.
Here's an example of the ternary operator in Java:
int x = 10;
int y = 5;
String result = (x > y) ? "x is greater than y" : "y is greater than x";
System.out.println(result);
In this example, the ternary operator is used to assign a value to the result
variable. The ternary operator has three parts:
- The condition:
(x > y)
- The true result:
"x is greater than y"
- The false result:
"y is greater than x"
The ternary operator evaluates the condition, and if it is true, it returns the true result. If it is false, it returns the false result. In this example, the condition x > y
is true, so the true result "x is greater than y"
is assigned to the result
variable.
The value of result
is then printed to the console, which outputs "x is greater than y".
The ternary operator can be a useful shorthand for simple if-else statements and can make your code more concise and readable. However, it should be used with care, as complex ternary expressions can be difficult to understand and maintain.
Conclusion
In conclusion, the if
statement is one of the fundamental control flow statements in Java, which allows you to execute certain code only if a particular condition is true. The if
statement can be used on its own, or in combination with else
, else if
, and nested if
statements, to create more complex conditional logic.
In addition to the basic if
statement, Java provides a number of other conditional statements, such as the ternary operator, which can be used as a shorthand for simple if-else statements.
Understanding how to use these different types of conditional statements is an important part of writing effective and maintainable Java code.