Java Student Project With Code And Examples
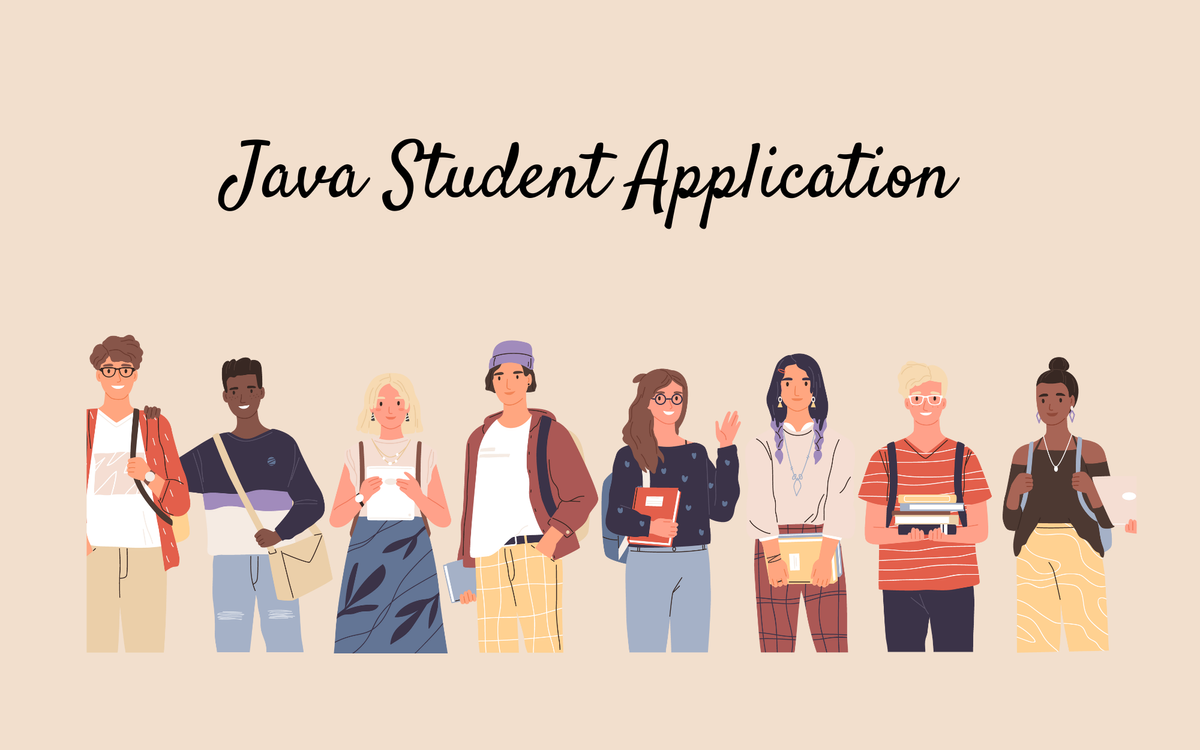
Let's create a simple Java student management system project. This project will allow you to add, view, and delete students from a list.
Step 1: Set Up Your Environment
Make sure you have Java installed on your computer.
Step 2: Create a New Java Class
Create a new Java class (e.g., Student.java
) to represent a student. This class will have attributes like id
, name
, and grade
.
public class Student {
private int id;
private String name;
private double grade;
public Student(int id, String name, double grade) {
this.id = id;
this.name = name;
this.grade = grade;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public double getGrade() {
return grade;
}
@Override
public String toString() {
return "Student ID: " + id + ", Name: " + name + ", Grade: " + grade;
}
}
Explanation:
- We have a
Student
class with three private attributes:id
,name
, andgrade
. - The constructor takes these attributes as parameters and initializes the object.
- There are getter methods to retrieve the student's
id
,name
, andgrade
. - The
toString
method provides a string representation of the student object.
Step 3: Create a Student Management System Class
Create another Java class (e.g., StudentManagementSystem.java
) for managing students.
import java.util.ArrayList;
import java.util.List;
public class StudentManagementSystem {
private List<Student> students;
public StudentManagementSystem() {
this.students = new ArrayList<>();
}
public void addStudent(Student student) {
students.add(student);
}
public void viewAllStudents() {
for (Student student : students) {
System.out.println(student);
}
}
public void deleteStudent(int id) {
students.removeIf(student -> student.getId() == id);
}
}
Explanation:
- We have a
StudentManagementSystem
class that contains a list of students. - The
addStudent
method allows us to add a student to the list. - The
viewAllStudents
method prints out details of all students in the list. - The
deleteStudent
method takes anid
as a parameter and removes the student with thatid
from the list.
Step 4: Test the Student Management System
Create a Main
class to test the student management system.
public class Main {
public static void main(String[] args) {
StudentManagementSystem system = new StudentManagementSystem();
Student student1 = new Student(1, "John Doe", 85.5);
Student student2 = new Student(2, "Jane Smith", 92.3);
system.addStudent(student1);
system.addStudent(student2);
system.viewAllStudents();
System.out.println("\nDeleting student with ID 1...\n");
system.deleteStudent(1);
system.viewAllStudents();
}
}
Explanation:
- In the
Main
class, we create aStudentManagementSystem
object. - We create two
Student
objects and add them to the system. - We view all students to see the list.
- We delete a student with ID 1 and view all students again to confirm the deletion.
Step 5: Run the Code
Compile and run the Java files. You should see the output showing the list of students and the student with ID 1 being deleted.
This is a simple student management system project. You can extend it by adding more functionality, like updating student information, searching for students, or persisting data to a file or database.