Java While Loop
In Java, the while loop is a control flow statement that allows a program to execute a block of code repeatedly while a given condition is true.
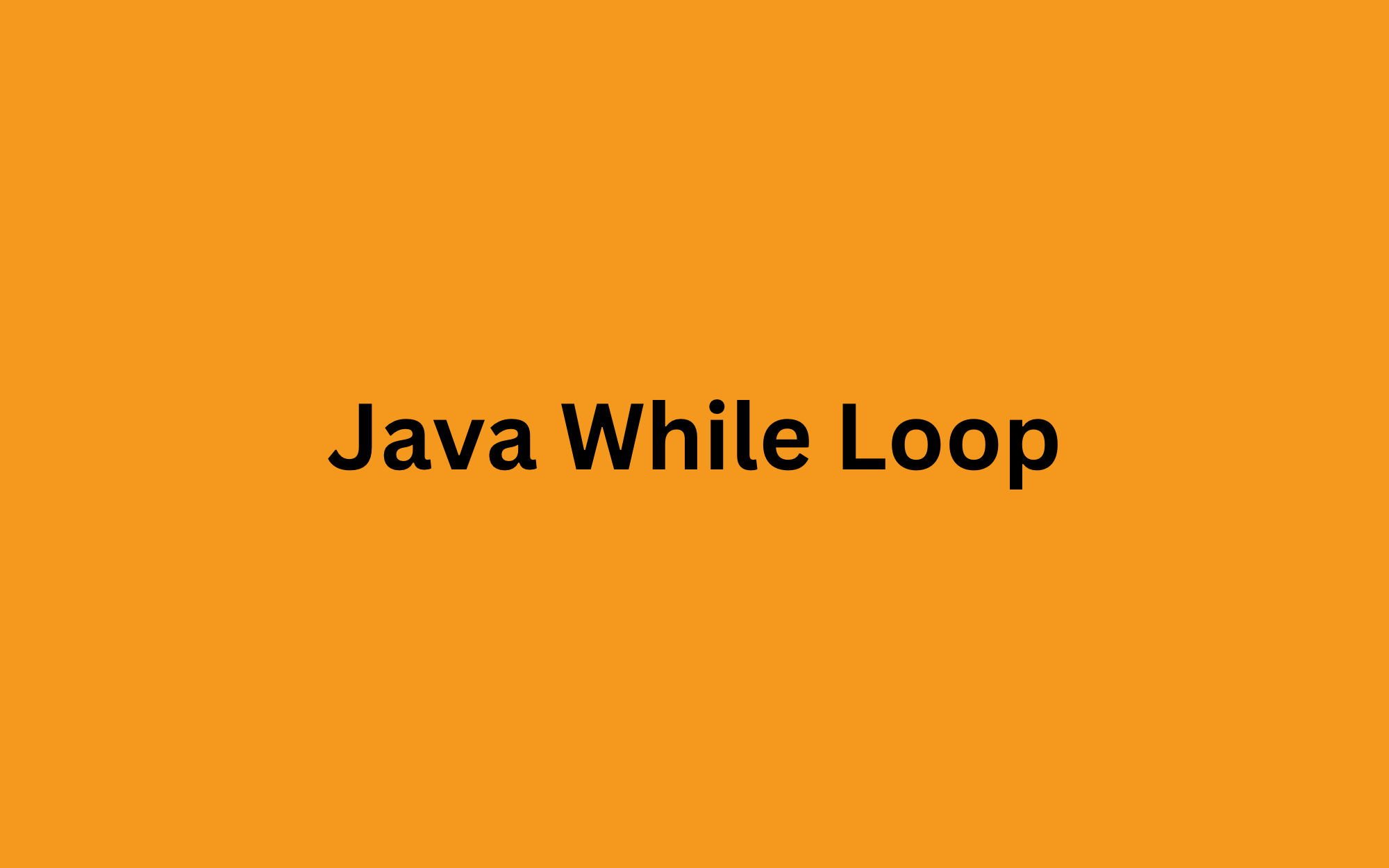
Introduction
In Java, the while
loop is a control flow statement that allows a program to execute a block of code repeatedly while a given condition is true. The while
loop is commonly used when the number of iterations is not known beforehand and is determined by the condition being evaluated.
Syntax and declaration
The syntax for a while
loop in Java is simple:
while (condition) {
// code to be executed while the condition is true
}
Here, condition
is an expression that is evaluated at the beginning of each iteration. If condition
is true, the code block inside the loop is executed. If condition
is false, the loop is skipped entirely, and the program continues with the code following the loop.
It's important to ensure that the condition
in a while
loop will eventually become false to avoid infinite looping. In cases where the condition
may never become false, such as in a program waiting for user input, a break
statement can be used to exit the loop manually.
The while
loop is a basic and commonly used loop in Java, and it's often used for tasks that require iteration until a certain condition is met, such as reading input or processing data.
Basic while-loop
Here's an example of a while
loop in Java:
int i = 1;
while (i <= 10) {
System.out.println(i);
i++;
}
In this example, the while
loop will iterate as long as i
is less than or equal to 10. The loop will execute the System.out.println(i)
statement, which will print the value of i
to the console, and then increment i
by 1 with the i++
statement.
The loop will repeat this process until i
reaches 11, at which point the loop will terminate and the program will continue with the code following the loop.
This example demonstrates how the while
loop can be used to iterate over a range of values and perform a certain action for each iteration, such as printing the value of i
in this case.
Using a sentinel value
Scanner scanner = new Scanner(System.in);
String input = "";
while (!input.equals("quit")) {
System.out.print("Enter a command (or 'quit' to exit): ");
input = scanner.nextLine();
System.out.println("You entered: " + input);
}
In this example, the while
loop continues until the user enters the word "quit". The loop prompts the user to enter a command and then prints out the user's input. The loop will continue to run until the user enters the word "quit", at which point the loop will terminate.
Infinite while-loop with a break statement
int i = 0;
while (true) {
if (i == 5) {
break;
}
System.out.println("Iteration " + i);
i++;
}
In this example, the while
loop is designed to iterate infinitely until i
reaches 5. The loop includes a conditional statement that checks if i
is equal to 5, and if so, it uses a break
statement to exit the loop. This technique can be useful in situations where it's not possible to know ahead of time how many iterations will be required.
Nested while-loops
int i = 0;
while (i < 5) {
int j = 0;
while (j < 5) {
System.out.print("*");
j++;
}
System.out.println();
i++;
}
In this example, the while
loop is nested inside another while
loop. The outer loop iterates five times, and the inner loop iterates five times for each iteration of the outer loop. The result is a block of asterisks printed to the console. This technique can be useful for complex tasks that require multiple levels of iteration.
Conclusion
In conclusion, the while
loop is a fundamental construct in Java that allows for repeated execution of code as long as a certain condition is true. It's a versatile loop that can be used for a wide range of tasks, from basic iteration to more complex control flow scenarios.
When using while
loops, it's important to ensure that the condition eventually becomes false to avoid infinite looping. Additionally, the break
and continue
statements can be used to modify the behavior of loops, allowing for greater control over program flow.
Overall, the while
loop is a powerful and flexible tool for implementing iterative processes in Java. Its simplicity and ease of use make it an essential part of any Java developer's toolkit.