Java LeetCode - Longest Substring Without Repeating Characters Solution
Java LeetCode - Longest Substring Without Repeating Characters Solution
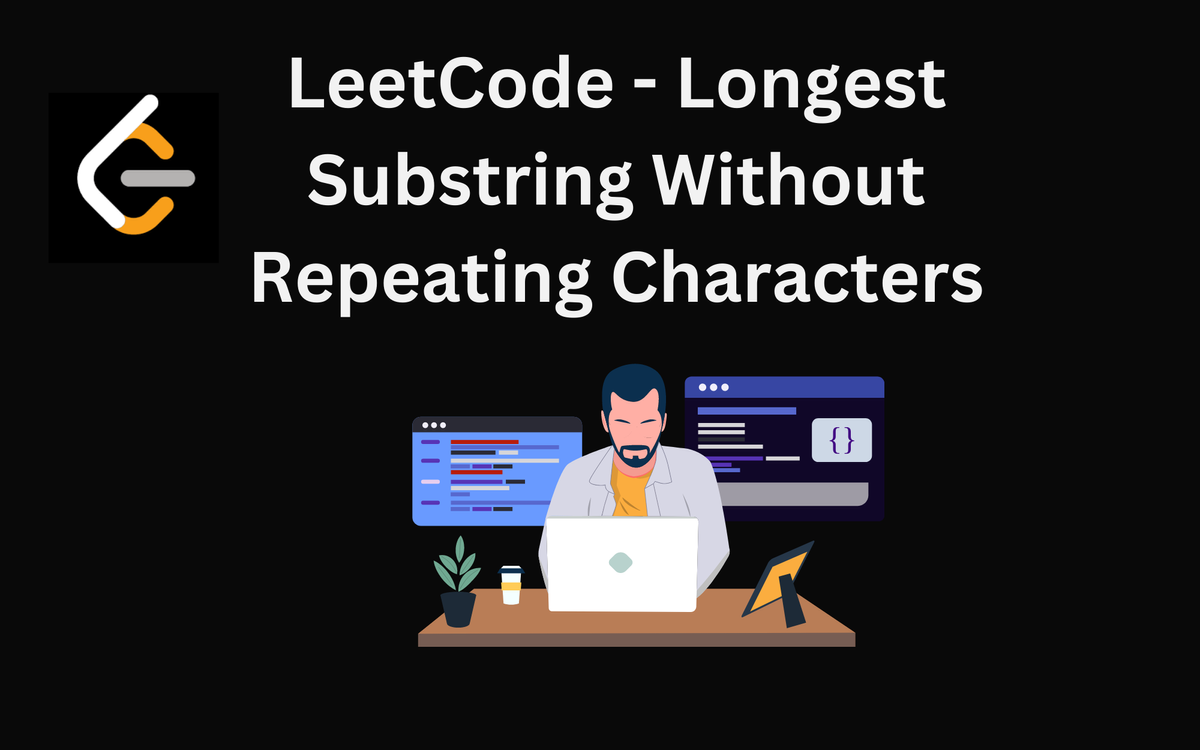
Given a string s
, find the length of the longest substring without repeating characters.
Example 1:
Input: s = "abcabcbb"
Output: 3
Explanation: The answer is "abc", with the length of 3.
Example 2:
Input: s = "bbbbb"
Output: 1
Explanation: The answer is "b", with the length of 1.
Example 3:
Input: s = "pwwkew"
Output: 3
Explanation: The answer is "wke", with the length of 3.
Notice that the answer must be a substring, "pwke" is a subsequence and not a substring.
Constraints:
- 0 <= s.length <= 5 X 104
- s consists of English letters, digits, symbols, and spaces.
Solution:
class Solution {
public int lengthOfLongestSubstring(String s) {
int[] chars = new int[2 << 6];
int start = 0;
int end = 0;
int res = 0;
while(end < s.length()) {
char ch = s.charAt(end);
chars[ch]++;
while(chars[ch] > 1) {
char removeChar = s.charAt(start);
chars[removeChar]--;
start++;
}
res = Math.max(res, end - start + 1);
end++;
}
return res;
}
}