Java Switch Statement
Switch statement is a control flow statement that allows program to execute different blocks of code depending on match.
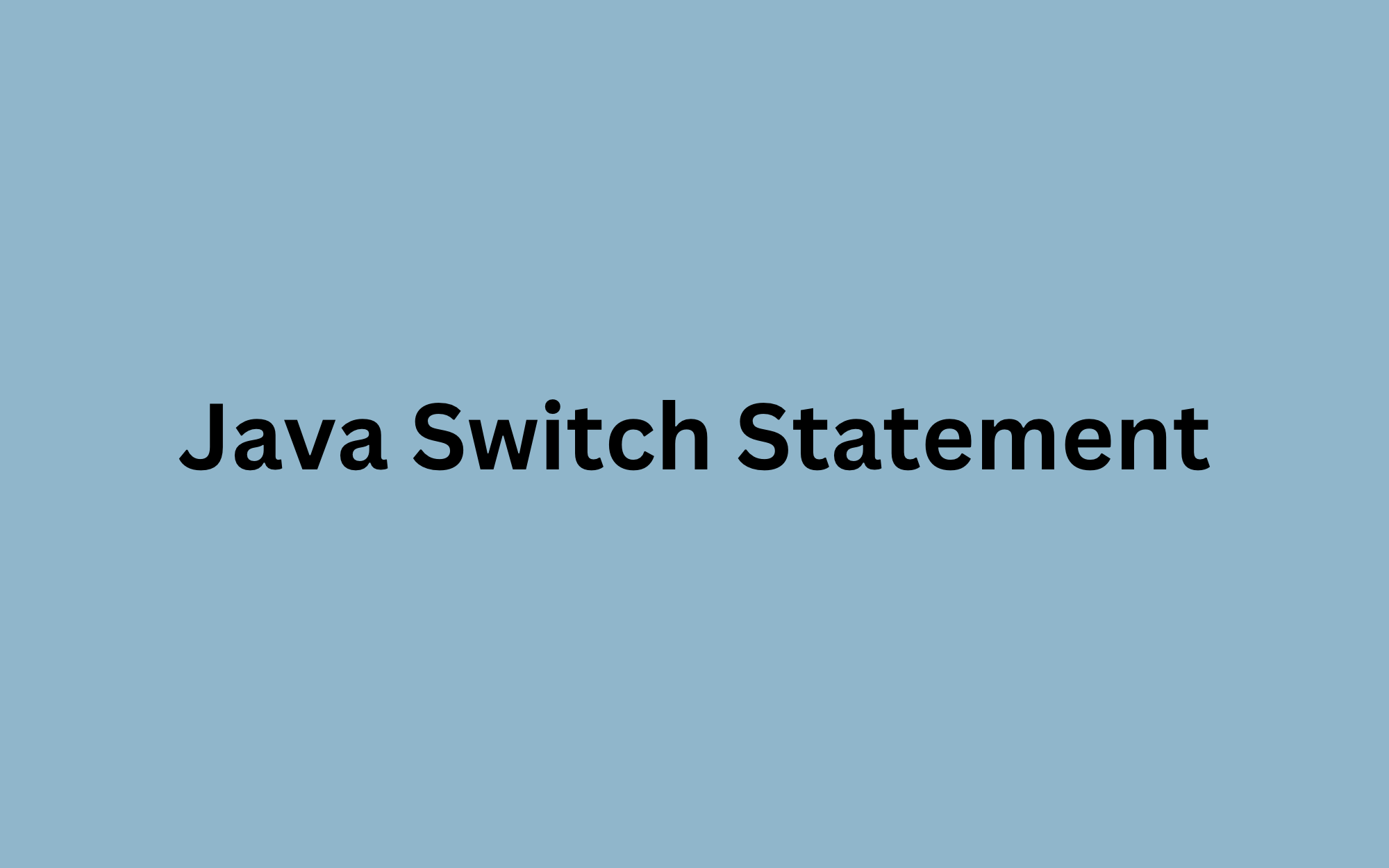
Introduction
In Java, the switch
statement is a control flow statement that allows a program to execute different blocks of code depending on the value of an expression. It is often used as a more concise alternative to an if-else
statement when comparing a single variable against multiple possible values.
The switch
statement evaluates the expression once and then compares it to the constant values specified in each case
statement. If the expression matches a case
value, the code block associated with that case
is executed. If none of the case
values match, the default
block is executed.
Overall, the switch
statement can provide a more streamlined and efficient way to execute different blocks of code based on the value of a single expression in Java.
Syntax and declaration
The syntax for a switch
statement in Java:
switch (expression) {
case value1:
// code to be executed if expression is equal to value1
break;
case value2:
// code to be executed if expression is equal to value2
break;
case value3:
// code to be executed if expression is equal to value3
break;
// more cases can be added here
default:
// code to be executed if expression doesn't match any case
}
Here, expression
is a variable or expression that is compared against the different case
values. If expression
is equal to a case
value, the corresponding block of code is executed. The break
statement is used to exit the switch
block and prevent the execution of subsequent cases.
If expression
does not match any of the case
values, the default
block of code is executed. The default
block is optional, but it is recommended to include it in order to handle unexpected or undefined input.
Note that the switch
statement can only be used with certain data types in Java, including byte
, short
, char
, int
, String
, and enum
types.
Types of switch statements
Here's an overview of basic to advanced switch
statements in Java.
Basic switch statment
The basic switch
statement compares a variable or expression to a set of constant values and executes the block of code corresponding to the matching value. It has the syntax I mentioned in my previous answer.
switch (expression) {
case value1:
// code to be executed if expression is equal to value1
break;
case value2:
// code to be executed if expression is equal to value2
break;
case value3:
// code to be executed if expression is equal to value3
break;
// more cases can be added here
default:
// code to be executed if expression doesn't match any case
}
Multiple cases
A switch
statement can have multiple case
labels that share the same block of code. For example:
switch (dayOfWeek) {
case 1:
case 2:
case 3:
case 4:
case 5:
System.out.println("Weekday");
break;
case 6:
case 7:
System.out.println("Weekend");
break;
default:
System.out.println("Invalid day");
}
In this example, if dayOfWeek
is 1, 2, 3, 4, or 5, the "Weekday" message is printed. If dayOfWeek
is 6 or 7, the "Weekend" message is printed.
Nested switch statements
A switch
statement can be nested inside another switch
statement to provide additional levels of branching.
For example:
switch (userInput) {
case "create":
switch (userObjectType) {
case "book":
// code to create a book object
break;
case "movie":
// code to create a movie object
break;
default:
// code to handle invalid object type
}
break;
case "delete":
// code to delete an object
break;
default:
// code to handle invalid input
}
In this example, the outer switch
statement handles the userInput
, and the inner switch
statement handles the userObjectType
for the "create" case.
Enhanced switch statements
Starting from Java 12, there is a new feature called "enhanced switch
statements" that allows for more concise and powerful switch
statements. It includes features such as support for case
expressions, arrow
syntax, and yield
statements.
For example:
int numLetters = switch (dayOfWeek) {
case "Monday", "Wednesday", "Friday" -> 6;
case "Tuesday", "Thursday", "Saturday" -> 7;
case "Sunday" -> 8;
default -> throw new IllegalStateException("Invalid day");
};
In this example, the enhanced switch
statement assigns a value to numLetters
based on the length of the day name. The arrow
syntax is used to define the case
expressions, and the yield
statement is used to return the value for each case.
These are some basic to advanced switch
statements in Java that can be used for different programming scenarios.
Conclusion
In conclusion, the switch
statement in Java provides a convenient and efficient way to branch the execution of code based on the value of an expression. It allows for concise and readable code by grouping related cases together and provides a default option for handling unexpected input.
When using a switch
statement, it's important to remember to include a break
statement to prevent the execution of subsequent cases and to include a default
block to handle unexpected input. Additionally, it's important to note that switch
statements can only be used with certain data types in Java.
Overall, the switch
statement is a useful tool in Java programming, and with its added features such as multiple cases, nested switches, and enhanced switch statements, it can be utilized in many different programming scenarios.